Unity SDK Quick Start Guide (SDK v3.0.0)
Overview
This section shows how to install the CleverTap SDK, track your first user event, and see this information within the CleverTap dashboard in less than ten minutes. CleverTap provides Unity SDK that enables app developers to track, segment, and engage their users.
Install
You can now install the CleverTap Unity SDK using the .unitypackage
Unity package or as a local package through Unity Package Manager (UPM).
This installation involves the following major steps:
- Import the CleverTap Unity Package
- Import the CleverTap Unity Package as a Local Dependency
- Set Up the Unity SDK
- Callbacks
Import the CleverTap Unity Package
- Download the latest version of the CleverTap Unity package. Import the
.unitypackage
into your Unity Project. Go to Assets > Import Package > Custom Package. - Add the PlayServiceResolver and the ExternalDependencyManager folders. These folders will install the EDM4U plugin, which automatically adds all the Android and iOS dependencies when building your project.
- Ensure that the
AndroidPostImport
script is added since it sets upclevertap-android-wrapper
library for Android.
Import the CleverTap Unity Package as a Local Dependency
Clone the latest release version of CleverTap Unity SDK. The SDK can be imported as a local package through the Unity Package Manager.
Set Up the Unity SDK
CleverTap API can be accessed anywhere in your project by simply calling the static CleverTap class. No need to create GameObject with the CleverTapUnity name or attach any script. The new architecture handles the following:
- Instantiation of platform-specific binding
- Creation of
GameObject
- Script attachment.
You can view your CleverTap Account ID
and CleverTap Account Token
from the Settings page.
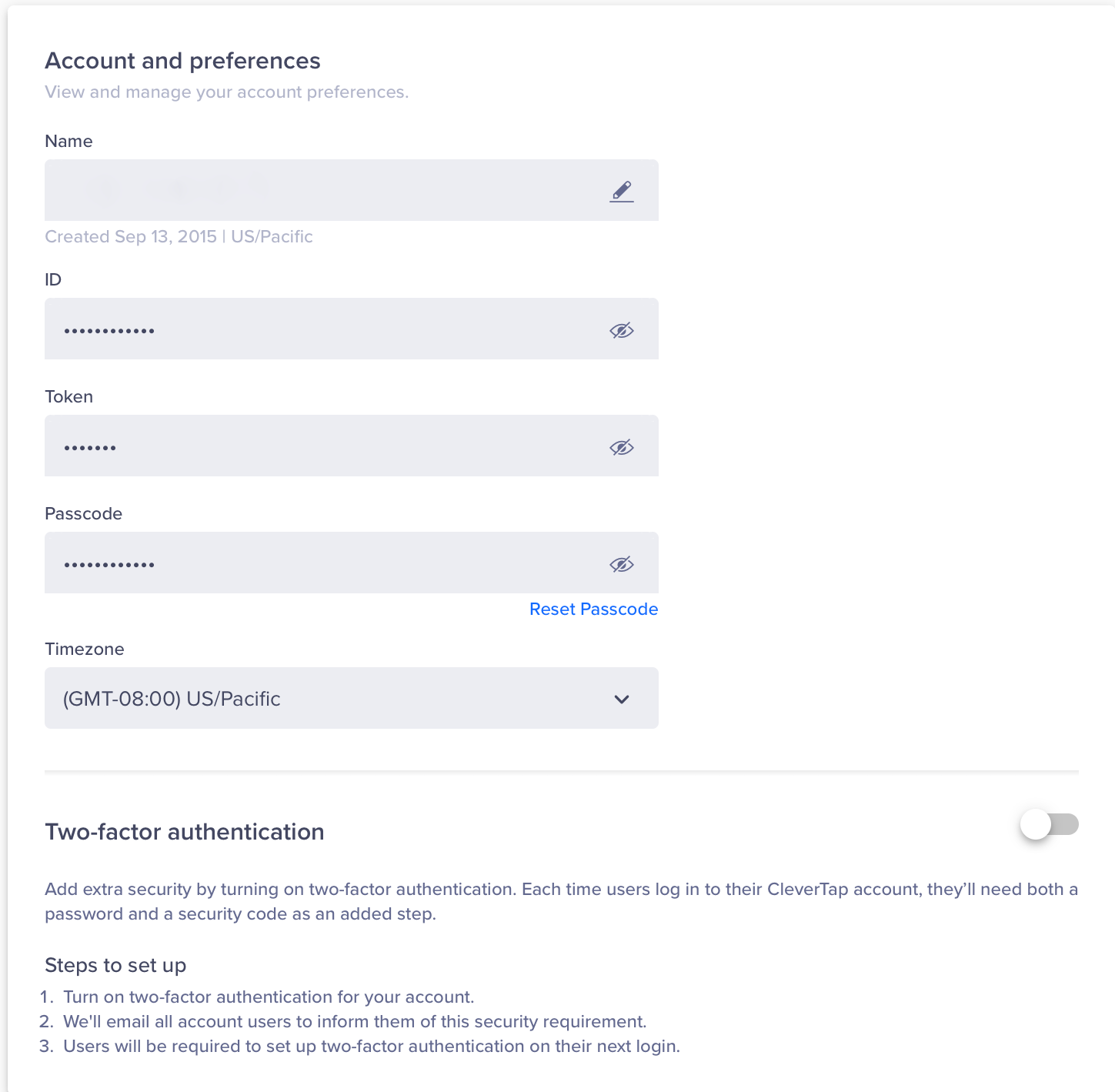
Add CleverTap Account and Preferences
// Initialize CleverTap
CleverTap.LaunchWithCredentialsForRegion({YOUR_CLEVERTAP_ACCOUNT_ID}, {YOUR_CLEVERTAP_ACCOUNT_TOKEN}, {CLEVERTAP_ACCOUNT_REGION});
// Enable personalization
CleverTap.EnablePersonalization();
Note
CleverTapBinding.cs
andCleveTapUnity.cs
are now obsolete. They will still be usable with minor changes but will be removed in the future.
Callbacks
A new mechanism to handle callbacks is introduced. You can now add an event listener for a callback directly through the CleverTap static events. No need to set all callbacks in the CleverTapUnity.cs
anymore.
CleverTap.OnCleverTapDeepLinkCallback += YOUR_CALLBACK_METHOD;
CleverTap.OnCleverTapProfileInitializedCallback += YOUR_CALLBACK_METHOD;
CleverTap.OnCleverTapProfileUpdatesCallback += YOUR_CALLBACK_METHOD;
This introduces breaking changes in the CleverTapUnity.cs
, rendering all existing callback methods obsolete, and these methods will not be called anymore. If you still want to use the callbacks through the CleverTapUnity.cs
methods, each method must subscribe to a new event listener found at CleverTap.On{CleveTapUnity_Callback_MethodName}
.
The following is a sample code on how to subscribe to new event listeners with existing methods in CleverTapUnity.cs
:
CleverTap.OnCleverTapDeepLinkCallback += CleverTapDeepLinkCallback;
CleverTap.OnCleverTapProfileInitializedCallback += CleverTapProfileInitializedCallback;
CleverTap.OnCleverTapProfileUpdatesCallback += CleverTapProfileUpdatesCallback;
CleverTap.OnCleverTapPushOpenedCallback += CleverTapPushOpenedCallback;
CleverTap.OnCleverTapInitCleverTapIdCallback += CleverTapInitCleverTapIdCallback;
CleverTap.OnCleverTapInAppNotificationDismissedCallback += CleverTapInAppNotificationDismissedCallback;
CleverTap.OnCleverTapInAppNotificationShowCallback += CleverTapInAppNotificationShowCallback;
CleverTap.OnCleverTapOnPushPermissionResponseCallback += CleverTapOnPushPermissionResponseCallback;
CleverTap.OnCleverTapInAppNotificationButtonTapped += CleverTapInAppNotificationButtonTapped;
CleverTap.OnCleverTapInboxDidInitializeCallback += CleverTapInboxDidInitializeCallback;
CleverTap.OnCleverTapInboxMessagesDidUpdateCallback += CleverTapInboxMessagesDidUpdateCallback;
CleverTap.OnCleverTapInboxCustomExtrasButtonSelect += CleverTapInboxCustomExtrasButtonSelect;
CleverTap.OnCleverTapInboxItemClicked += CleverTapInboxItemClicked;
CleverTap.OnCleverTapNativeDisplayUnitsUpdated += CleverTapNativeDisplayUnitsUpdated;
CleverTap.OnCleverTapProductConfigFetched += CleverTapProductConfigFetched;
CleverTap.OnCleverTapProductConfigActivated += CleverTapProductConfigActivated;
CleverTap.OnCleverTapProductConfigInitialized += CleverTapProductConfigInitialized;
CleverTap.OnCleverTapFeatureFlagsUpdated += CleverTapFeatureFlagsUpdated;
iOS Configurations
iOS dependencies are added using CocoaPods Podfile. After exporting the project to XCode, you need to run pod install
. This can be automated by EDM4U plugin to be done on each export. You can always manually install pods by running pod install
in the exported XCode project. Ensure to start .xcworkspace
to build the game with dependencies.
- Set your account ID, token, region, personalization, and IDFV settings. Go to Assets > CleverTap Settings
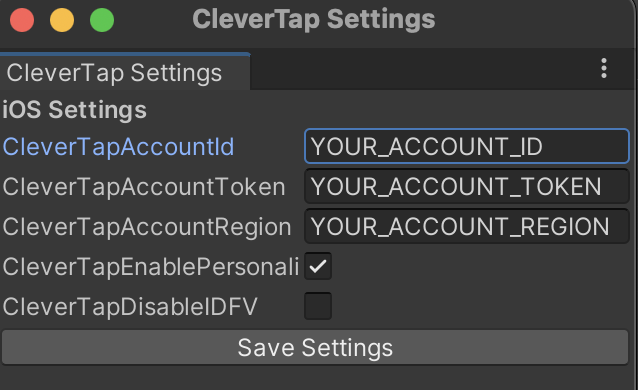
This is needed so the CleverTap Post Processor script can add those settings automatically in the Info.plist
file.
IDFV Usage for CleverTap ID
Starting from CleverTap Unity SDK 2.1.2, you can optionally disable the usage of IDFV for CleverTap ID. We recommend this for apps that send data from different iOS apps to a common CleverTap account. By default, the CleverTapDisableIDFV checkbox is cleared.
- Set up EDM4U plugin to install CocoaPods automatically. Go to Assets > External Dependency Manager > iOS Resolver > Settings. You can also install manually using the
pod install
.
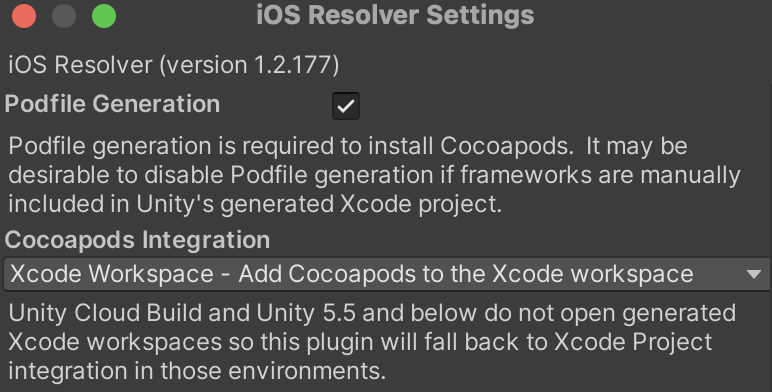
- Open the
.xcworkspace
project. - To enable Push Notifications, ensure to add the Push Notifications capability to your Xcode project.
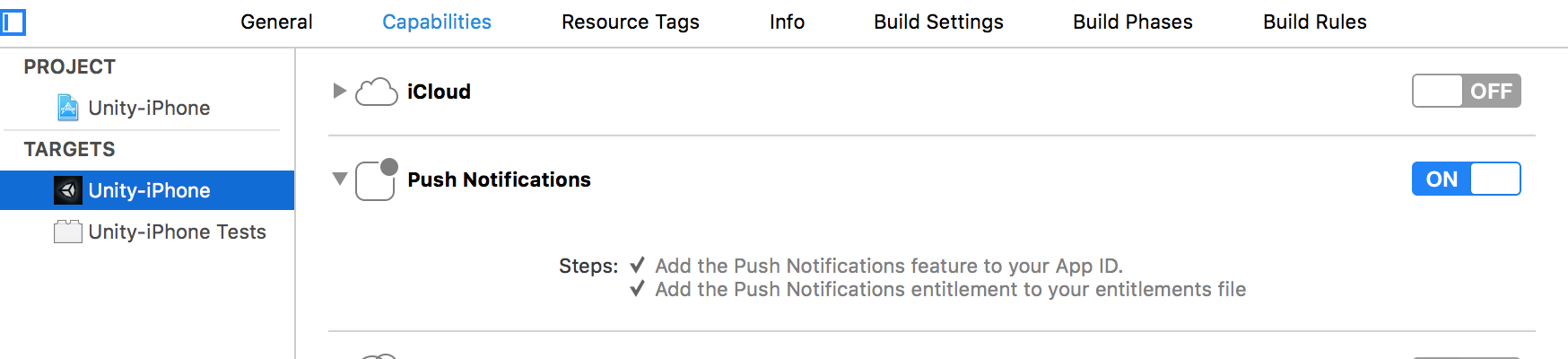
Enable Push Notifications
- Build and run your iOS project.
Android Configurations
- Go to File > Build Settings > Android > Player Settings > Publishing Settings > Build. Enable .gradle templates and custom AndroidManifest. EDM4U populates the Custom Main Gradle Template and Gradle Properties Template with the required Android dependencies.
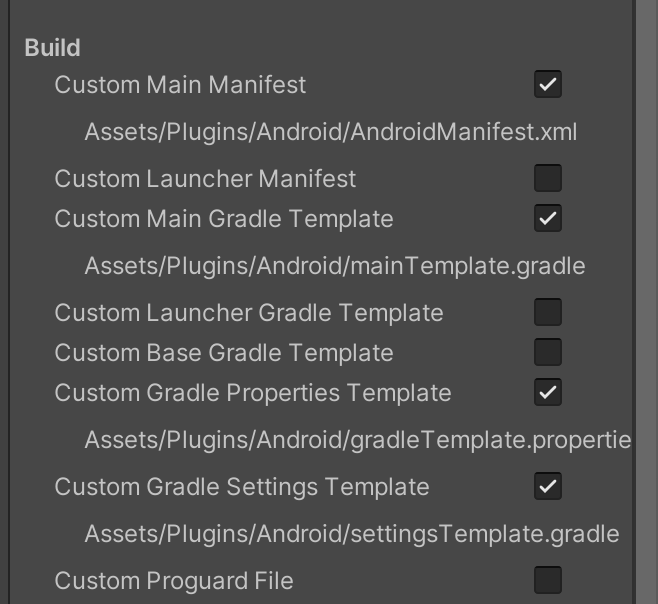
- Once you enable the custom Android Manifest, ensure to add additional configurations to enable Push Notifications and override the default
UnityActivity
. Add your Bundle Identifier, FCM Sender ID, CleverTap Account ID, CleverTap Token, and Deep Link URL scheme (if applicable):
<?xml version="1.0" encoding="utf-8"?>
<manifest package="YOUR_BUNDLE_IDENTIFIER"
xmlns:android="http://schemas.android.com/apk/res/android"
android:installLocation="preferExternal"
android:versionCode="1"
android:versionName="1.0">
<supports-screens
android:anyDensity="true"
android:largeScreens="true"
android:normalScreens="true"
android:smallScreens="true"
android:xlargeScreens="true" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="com.google.android.c2dm.permission.RECEIVE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<uses-feature android:glEsVersion="0x00020000" />
<uses-feature
android:name="android.hardware.touchscreen"
android:required="false" />
<uses-feature
android:name="android.hardware.touchscreen.multitouch"
android:required="false" />
<uses-feature
android:name="android.hardware.touchscreen.multitouch.distinct"
android:required="false" />
<application
android:debuggable="true"
android:icon="@drawable/app_icon"
android:isGame="true"
android:label="@string/app_name"
android:theme="@style/UnityThemeSelector">
<activity
android:name="com.clevertap.unity.CleverTapOverrideActivity"
android:configChanges="mcc|mnc|locale|touchscreen|keyboard|keyboardHidden|navigation|orientation|screenLayout|uiMode|screenSize|smallestScreenSize|fontScale"
android:label="@string/app_name"
android:launchMode="singleTask"
android:screenOrientation="fullSensor">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
<category android:name="android.intent.category.LEANBACK_LAUNCHER" />
</intent-filter>
<!-- Deep Links uncomment and replace YOUR_URL_SCHEME, if applicable, or remove if not supporting deep links-->
<!--
<intent-filter android:label="@string/app_name">
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="YOUR_URL_SCHEME" />
</intent-filter>
-->
<meta-data
android:name="unityplayer.UnityActivity"
android:value="true" />
</activity>
<service
android:name="com.clevertap.android.sdk.pushnotification.fcm.FcmMessageListenerService"
android:exported="true">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
<service
android:name="com.clevertap.android.sdk.pushnotification.CTNotificationIntentService"
android:exported="false">
<intent-filter>
<action android:name="com.clevertap.PUSH_EVENT" />
</intent-filter>
</service>
<meta-data
android:name="FCM_SENDER_ID"
android:value="id:YOUR_FCM_SENDER_ID" />
<meta-data
android:name="CLEVERTAP_ACCOUNT_ID"
android:value="Your CleverTap Account ID" />
<meta-data
android:name="CLEVERTAP_TOKEN"
android:value="Your CleverTap Account Token" />
</application>
</manifest>
- Add your
google-services.json
file to the project's Assets folder. - Build your app or Android project.
Initialize CleverTap SDK
// Initialize CleverTap
CleverTap.LaunchWithCredentialsForRegion({YOUR_CLEVERTAP_ACCOUNT_ID}, {YOUR_CLEVERTAP_ACCOUNT_TOKEN}, {CLEVERTAP_ACCOUNT_REGION});
// Enable personalization
CleverTap.EnablePersonalization();
Track User Profiles
Create a User profile when the user logs in (On User Login):
Dictionary<string, string> newProps = new Dictionary<string, string>();
newProps.Add("email", "[email protected]");
newProps.Add("Identity", "123456");
newProps.put("Name", "Jack Montana"); // String
newProps.put("Identity", "61026032"); // String
newProps.put("Email", "[email protected]"); // Email address of the user
newProps.put("Phone", "+14155551234"); // Phone (with the country code, starting with +)
newProps.put("Gender", "M"); // Can be either M or F
CleverTap.OnUserLogin(newProps);
Track User Events
Record an event without event data:
// record basic event with no properties
CleverTap.RecordEvent("testEvent");
Record an event with event data:
// record event with properties
Dictionary<string, object> Props = new Dictionary<string, object>();
Props.Add("testKey", "testValue");
CleverTap.RecordEvent("testEventWithProps", Props);
Updated 5 months ago