Cordova Quick Start Guide
Steps to Install
Grab the Account ID and Token values from the Settings page of your CleverTap Dashboard.
Cordova Integration
Follow the steps below to get started with SDK integration for your Cordova app:
Step 1: Add the Cordova plugin using the following command in your command line interface:
cordova plugin add https://github.com/CleverTap/clevertap-cordova.git --variable CLEVERTAP_ACCOUNT_ID="YOUR CLEVERTAP ACCOUNT ID" --variable CLEVERTAP_TOKEN="YOUR CLEVERTAP ACCOUNT TOKEN"
Step 2: Add the following XML tags to your www/config.xml
file.
www/config.xml
file.<preference name="android-build-tool" value="gradle" />
<gap:plugin name="clevertap-cordova" source="npm">
<param name="CLEVERTAP_ACCOUNT_ID" value="YOUR CLEVERTAP ACCOUNT ID" />
<param name="CLEVERTAP_TOKEN" value="YOUR CLEVERTAP ACCOUNT TOKEN" />
<param name="GCM_PROJECT_NUMBER" value="YOUR GCM PROJECT NUMBER" /> // for v1.2.5 and lower of the plugin
</gap:plugin>
Step 3: Add CleverTap.notifyDeviceReady();
to your onDeviceReady
callback in www/js/index.js
CleverTap.notifyDeviceReady();
to your onDeviceReady
callback in www/js/index.js
onDeviceReady: function() {
app.receivedEvent('deviceready');
CleverTap.notifyDeviceReady();
...
},
Ionic/Capacitor Integration
Follow the steps below to start SDK integration for your Ionic or Capacitor app:
Step 1: Add the Ionic Cordova or Capacitor plugin using the following commands:
// Install Ionic Native TypeScript wrapper for CleverTap
npm i @awesome-cordova-plugins/clevertap
// Install Cordova plugin for CleverTap
ionic cordova plugin add https://github.com/CleverTap/clevertap-cordova.git --variable CLEVERTAP_ACCOUNT_ID="YOUR CLEVERTAP ACCOUNT ID" --variable CLEVERTAP_TOKEN="YOUR CELVERTAP ACCOUNT TOKEN"
Step 2: Add CleverTap as a provider in your App module:
import { CleverTap } from '@ionic-native/clevertap/ngx';
export class AppComponent {
constructor(platform: Platform, statusBar: StatusBar, splashScreen: SplashScreen, clevertap: CleverTap) {
platform.ready().then(() => {
// once the platform is ready and plugins are available,
// do all higher level native things
clevertap.notifyDeviceReady();
}
}
iOS
Check your .plist file for the added Account ID and Account Token.
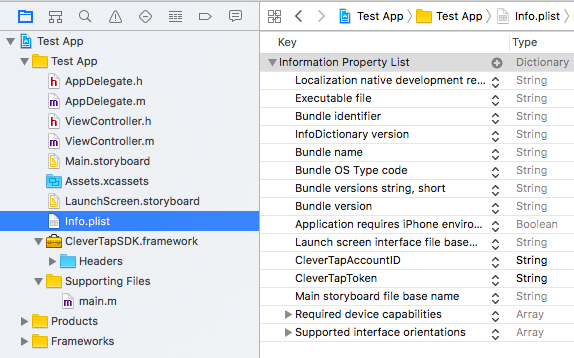
Information Property List-Info.plist File
Add Region Code
To know how to add region code for iOS in Cordova SDK, refer to Region Codes.
Android
Ensure that the following metadata tags are added inside the tags of your AndroidManifest.xml
:
<meta-data
android:name="CLEVERTAP_ACCOUNT_ID"
android:value="Your CleverTap Project ID"/>
<meta-data
android:name="CLEVERTAP_TOKEN"
android:value="Your CleverTap Project Token"/>
Add Region Code
To know how to add region code for Android in Cordova SDK, refer to Region Codes.
Set Lifecycle Callback
You can add CleverTapβs class as your application class in AndroidManifest.xml
file to capture system events correctly.
<application
android:name="com.clevertap.android.sdk.Application">
The above step is extremely important and enables CleverTap to track notification opens, display in-app notifications, track deep links, and other important user behavior.
Add Permissions
Ensure to request the following permissions for your app:
<!-- Required to allow the app to send events -->
<uses-permission android:name="android.permission.INTERNET"/>
<!-- Recommended so that we can be smart about when to send the data -->
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permission android:name="android.permission.WAKE_LOCK" />
Refer to the example AndroidManifest.xml.
Add Dependencies
Check that your build.gradle
file includes the play-services and support library dependencies:
Migrating from ExoPlayer to AndroidX Media3
Starting from CleverTap Cordova SDK v3.3.0, CleverTap now supports AndroidX Media3, replacing the deprecated ExoPlayer libraries. While CleverTap continues to support ExoPlayer.
For a smoother migration to AndroidX Media3. Update the following dependencies:
implementation fileTree(dir: 'libs', include: '*.jar')
debugCompile(project(path: "CordovaLib", configuration: "debug"))
releaseCompile(project(path: "CordovaLib", configuration: "release"))
// SUB-PROJECT DEPENDENCIES START
implementation "com.google.firebase:firebase-core:+"
implementation "com.google.firebase:firebase-messaging:23.0.6"
implementation 'androidx.core:core:1.3.0'
implementation 'androidx.fragment:fragment:1.3.6'
implementation "com.android.installreferrer:installreferrer:2.2" //Mandatory for v2.1.8 and above
// MANDATORY for App Inbox
implementation 'androidx.appcompat:appcompat:1.3.1'
implementation 'androidx.recyclerview:recyclerview:1.2.1'
implementation 'androidx.viewpager:viewpager:1.0.0'
implementation 'com.google.android.material:material:1.4.0'
implementation 'com.github.bumptech.glide:glide:4.12.0'
// Optional ExoPlayer Libraries for Audio/Video Inbox Messages. Audio/Video messages will be dropped without these dependencies
implementation 'com.google.android.exoplayer:exoplayer:2.19.1'
implementation 'com.google.android.exoplayer:exoplayer-hls:2.19.1'
implementation 'com.google.android.exoplayer:exoplayer-ui:2.19.1'
implementation fileTree(dir: 'libs', include: '*.jar')
debugCompile(project(path: "CordovaLib", configuration: "debug"))
releaseCompile(project(path: "CordovaLib", configuration: "release"))
// SUB-PROJECT DEPENDENCIES START
implementation "com.google.firebase:firebase-core:+"
implementation "com.google.firebase:firebase-messaging:23.0.6"
implementation 'androidx.core:core:1.3.0'
implementation 'androidx.fragment:fragment:1.3.6'
implementation "com.android.installreferrer:installreferrer:2.2" //Mandatory for v2.1.8 and above
// MANDATORY for App Inbox
implementation 'androidx.appcompat:appcompat:1.3.1'
implementation 'androidx.recyclerview:recyclerview:1.2.1'
implementation 'androidx.viewpager:viewpager:1.0.0'
implementation 'com.google.android.material:material:1.4.0'
implementation 'com.github.bumptech.glide:glide:4.12.0'
// Optional AndroidX Media3 Libraries for Audio/Video Inbox Messages. Audio/Video messages will be dropped without these dependencies
implementation "androidx.media3:media3-exoplayer:1.1.1"
implementation "androidx.media3:media3-exoplayer-hls:1.1.1"
implementation "androidx.media3:media3-ui:1.1.1"
Support AndroidX
To support AndroidX libraries, add the following to your config.xml
file -
<preference name="AndroidXEnabled" value="true" />
Integrate Javascript with CleverTap's Cordova Plugin
After integrating, all calls to the CleverTap SDK must be made from your Javascript.
Add the following listeners to your Javascript:
document.addEventListener('deviceready', this.onDeviceReady, false);
document.addEventListener('onCleverTapProfileSync', this.onCleverTapProfileSync, false); // optional: to be notified of CleverTap user profile synchronization updates
document.addEventListener('onCleverTapProfileDidInitialize', this.onCleverTapProfileDidInitialize, false); // optional, to be notified when the CleverTap user profile is initialized
document.addEventListener('onCleverTapInAppNotificationDismissed', this.onCleverTapInAppNotificationDismissed, false); // optional, to be receive a callback with custom in-app notification click data
document.addEventListener('onDeepLink', this.onDeepLink, false); // optional, register to receive deep links.
document.addEventListener('onPushNotification', this.onPushNotification, false); // optional, register to receive push notification payloads.
document.addEventListener('onCleverTapInboxDidInitialize', this.onCleverTapInboxDidInitialize, false); // optional, to check if CleverTap Inbox intialized
document.addEventListener('onCleverTapInboxMessagesDidUpdate', this.onCleverTapInboxMessagesDidUpdate, false); // optional, to check if CleverTap Inbox Messages were updated
document.addEventListener('onCleverTapInboxButtonClick', this.onCleverTapInboxButtonClick, false); // optional, to check if Inbox button was clicked with custom payload
document.addEventListener('onCleverTapInAppButtonClick', this.onCleverTapInAppButtonClick, false); // optional, to check if InApp button was clicked with custom payload
document.addEventListener('onCleverTapFeatureFlagsDidUpdate', this.onCleverTapFeatureFlagsDidUpdate, false); // optional, to check if Feature Flags were updated
document.addEventListener('onCleverTapProductConfigDidInitialize', this.onCleverTapProductConfigDidInitialize, false); // optional, to check if Product Config was initialized
document.addEventListener('onCleverTapProductConfigDidFetch', this.onCleverTapProductConfigDidFetch, false); // optional, to check if Product Configs were updated
document.addEventListener('onCleverTapProductConfigDidActivate', this.onCleverTapProductConfigDidActivate, false); // optional, to check if Product Configs were activated
document.addEventListener('onCleverTapDisplayUnitsLoaded', this.onCleverTapDisplayUnitsLoaded, false); // optional, to check if Native Display units were loaded
// deep link handling
onDeepLink: function(e) {
console.log(e.deeplink);
},
// push notification data handling
onPushNotification: function(e) {
console.log(JSON.stringify(e.notification));
},
onCleverTapInboxDidInitialize: function() {
CleverTap.showInbox({"navBarTitle":"My App Inbox","tabs": ["tag1", "tag2"],"navBarColor":"#FF0000"});
},
onCleverTapInboxMessagesDidUpdate: function() {
CleverTap.getInboxMessageUnreadCount(function(val) {console.log("Inbox unread message count"+val);})
CleverTap.getInboxMessageCount(function(val) {console.log("Inbox read message count"+val);});
},
onCleverTapInAppButtonClick: function(e) {
console.log("onCleverTapInAppButtonClick");
console.log(e.customExtras);
},
onCleverTapInboxButtonClick: function(e) {
console.log("onCleverTapInboxButtonClick");
console.log(e.customExtras);
},
onCleverTapFeatureFlagsDidUpdate: function() {
console.log("onCleverTapFeatureFlagsDidUpdate");
},
onCleverTapProductConfigDidInitialize: function() {
console.log("onCleverTapProductConfigDidInitialize");
},
onCleverTapProductConfigDidFetch: function() {
console.log("onCleverTapProductConfigDidFetch");
},
onCleverTapProductConfigDidActivate: function() {
console.log("onCleverTapProductConfigDidActivate");
},
onCleverTapDisplayUnitsLoaded: function(e) {
console.log("onCleverTapDisplayUnitsLoaded");
console.log(e.units);
},
Track User Profiles
Create a user profile (onUserLogin
method):
var stuff = ["bags", "shoes"];
var profile = {
'Name': 'Captain America',
'Identity': '100',
'Email': '[email protected]',
'Phone': '+14155551234',
'stuff': stuff
}
CleverTap.onUserLogin(profile);
Track User Events
Record an event with event data:
var eventData = {
// Key: Value
'first': 'partridge',
'second': 'turtledoves'
}
CleverTap.recordEventWithName('Cordova Event', eventData);
Record an event without event data:
CleverTap.recordEventWithName('Cordova Event');
Updated 3 months ago