Unity Push Notifications
Push Notifications
Note
If you are using Unity SDK v3.0.0 or higher, use
Clevertap
instead ofClevertapBindings
method.
Android
To use Push Notifications out of the box using CleverTap, add the following entries to your AndroidManifest.xml
.
<application>
....
....
<service android:name="com.clevertap.android.sdk.pushnotification.fcm.FcmMessageListenerService">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
</application>
Create Notification Channel
CleverTapBinding.CreateNotificationChannel("YourChannelId", "Your Channel Name", "Your Channel Description", 5, true);
Delete Notification Channel
CleverTapBinding.DeleteNotificationChannel("YourChannelId");
Create a Group Notification Channel
CleverTapBinding.CreateNotificationChannelGroup("YourGroupId", "Your Group Name");
Delete a Group Notification Channel
CleverTapBinding.DeleteNotificationChannelGroup("YourGroupId");
Create Custom Notification Icon
To set a custom notification icon (only for the small icon), add the following metadata entry in your AndroidManifest.xml.
<meta-data
android:name="CLEVERTAP_NOTIFICATION_ICON"
android:value="ic_stat_red_star"/> <!-- name of your file in the drawable directory without the file extension. -->
For more information about setting a small notification icon, refer to the Set the Small Notification Icon section.
iOS
To set up and register for push notifications, proceed as follows:
- Set up push notifications for your app.
- Call the following from your CSS file.
CleverTapBinding.RegisterPush();
Push Primer
A Push Primer explains the need for push notifications to your users and helps to improve your engagement rates. It is an InApp notification that you can show to your users before requesting notification permission.
Push Primer helps with the following:
- Allows you to educate your users on why you are asking for this permission before invoking a system dialog to seek user permission.
- Acts as a precursor to the complicated system dialog and thus allows you to seek permission multiple times if previously denied without making your users search the device settings.
CleverTap Unity SDK supports push primer for push notifications starting with the v2.4.0 release.
iOS and Android Platform Compatibility for Push Primer
- The minimum supported version for the iOS platform is 10.0.
- The minimum supported version for the Android platform is Android 13.
The following are the two ways to handle the new push notification changes:
Push Primer using Half Interstitial Local InApp
You can send a customized push primer notification with an image, text, and button using this template. You can also modify the notification's text, button, and background color.
Refer to the following images for Push Primer using Half-Interstitial InApp Template:
- Android
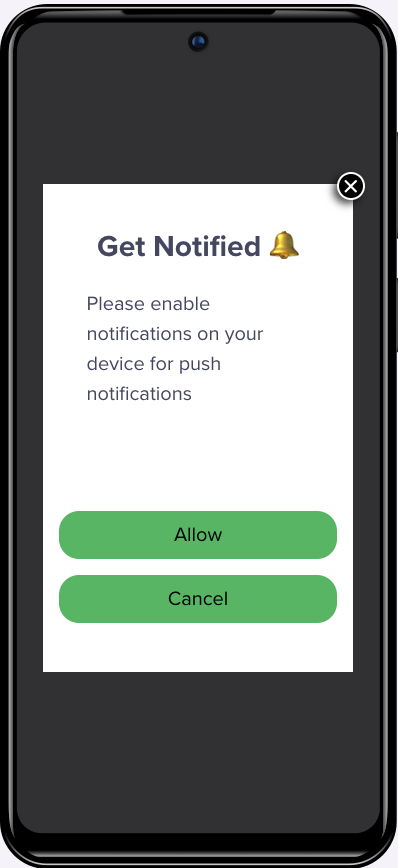
Push Primer Using Half-Interstitial InApp Template in Android
- iOS
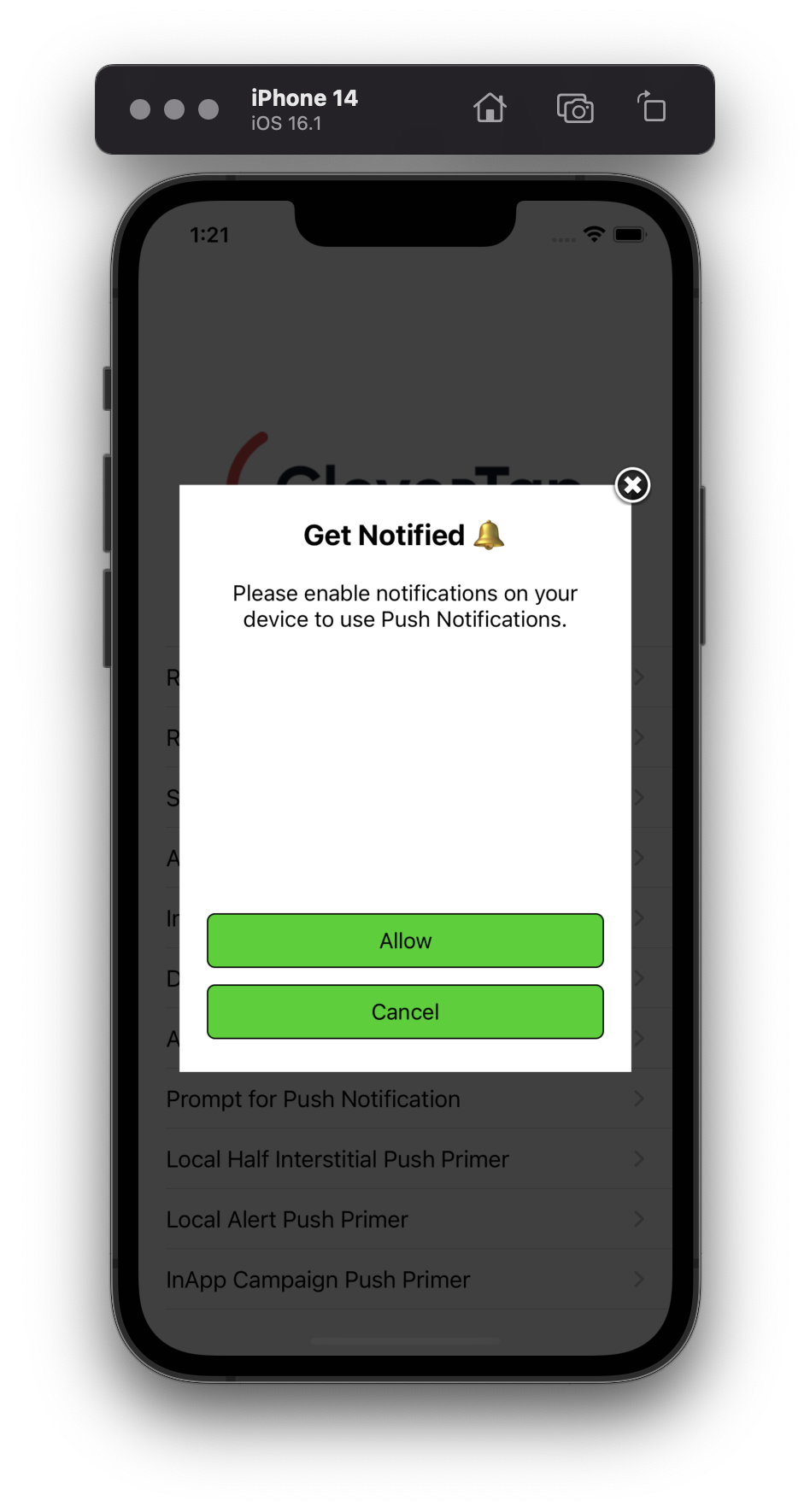
Push Primer Using Half-Interstitial InApp Template in iOS
Add the following code to your C# file to create Push Primer using the Half-Interstitial InApp template:
Dictionary<string, object> item = new Dictionary<string, object>();
item.Add("inAppType", "half-interstitial");
item.Add("titleText", "Get Notified");
item.Add("messageText", "Please enable notifications on your device to use Push Notifications.");
item.Add("followDeviceOrientation", true);
item.Add("positiveBtnText", "Allow");
item.Add("negativeBtnText", "Cancel");
item.Add("backgroundColor", "#FFFFFF");
item.Add("btnBorderColor", "#0000FF");
item.Add("titleTextColor", "#0000FF");
item.Add("messageTextColor", "#000000");
item.Add("btnTextColor", "#FFFFFF");
item.Add("btnBackgroundColor", "#0000FF");
item.Add("imageUrl", "https://icons.iconarchive.com/icons/treetog/junior/64/camera-icon.png");
item.Add("btnBorderRadius", "2");
item.Add("fallbackToSettings", true);
CleverTapBinding.PromptPushPrimer(item);
Push Primer using Alert Local InApp
You can create a basic push primer notification with a title, message, and two buttons using this template.
Refer to the following images for Push Primer using the Alert InApp template:
- Android
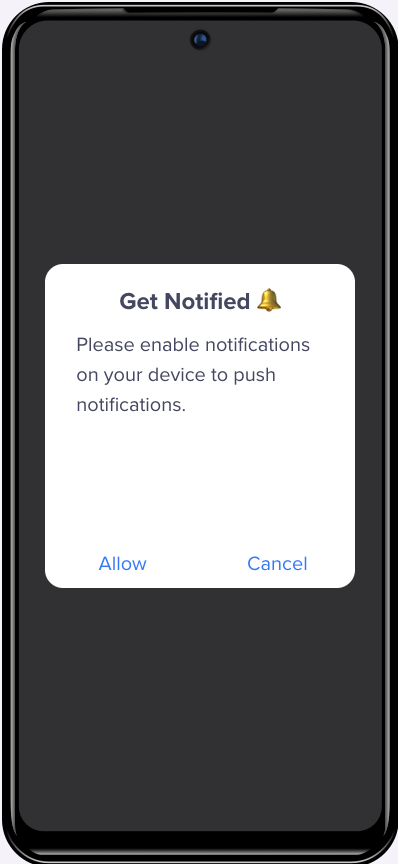
Push Primer using Alert InApp Template in Android
- iOS
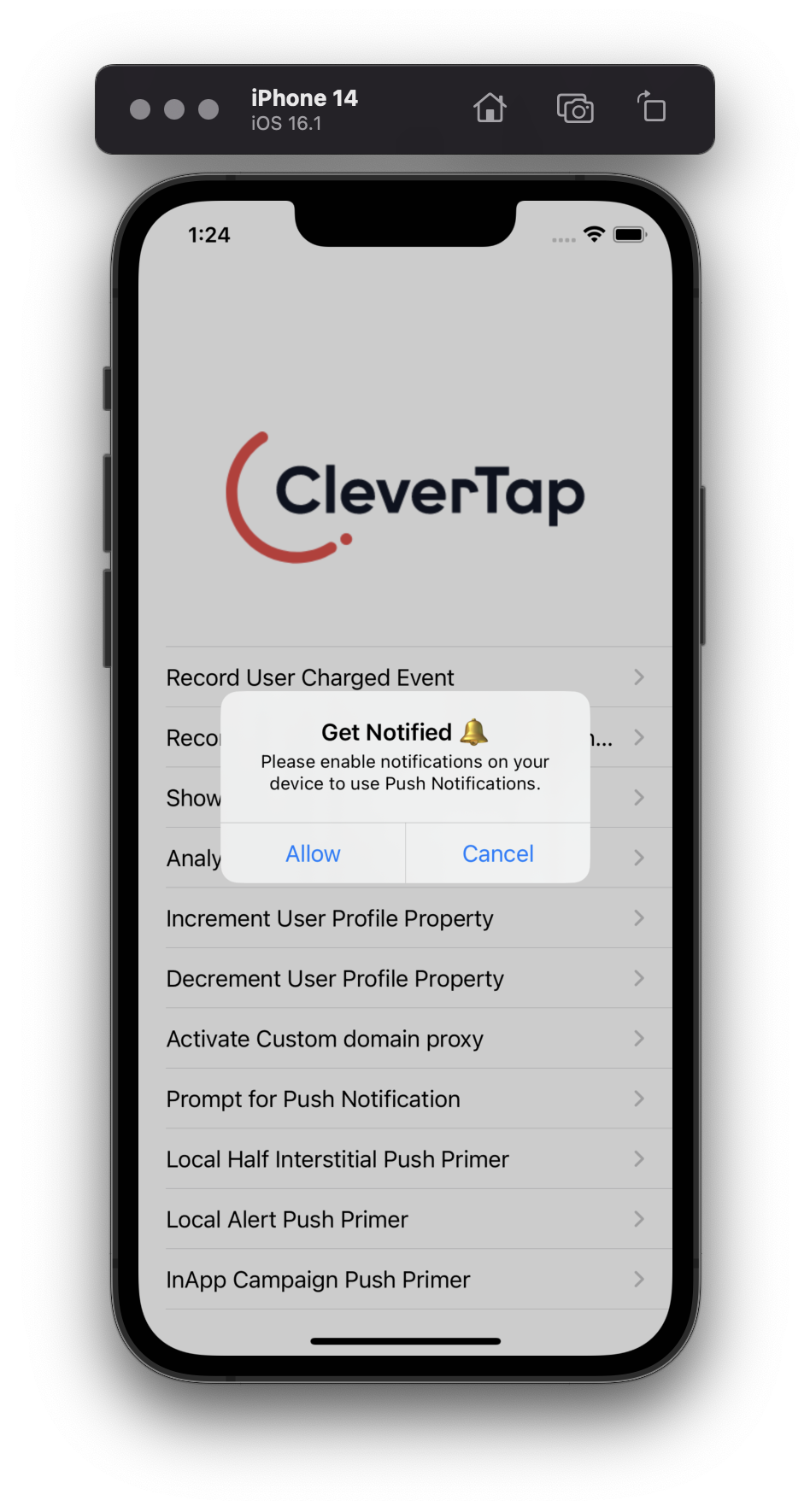
Push Primer using Alert InApp Template in iOS
Add the following code to your C# file to create a Push Primer using the Alert InApp template:
Dictionary<string, object> item = new Dictionary<string, object>();
item.Add("inAppType", "alert");
item.Add("titleText", "Get Notified");
item.Add("messageText", "Please enable notifications on your device to use Push Notifications.");
item.Add("followDeviceOrientation", true);
item.Add("fallbackToSettings", true);
CleverTapBinding.PromptPushPrimer(item);
Invoke Notification Permission Dialog without Push Primer
You can call the push permission dialogue directly without a Push Primer using the PromptForPushPermission(boolean)
method. It takes a boolean value as a parameter.
- If the value is set to true and permission is denied, then the user is redirected to the appβs notification settings.
- If the value is set to false, the callback is sent stating that the permission is denied.
CleverTapBinding.PromptForPushPermission(false);
Check the Push Notification Permission Status
The IsPushPermissionGranted
method can be used to check the status of the push notification permission for your application. The method returns the status of the push permission.
bool isPushPermissionGranted = CleverTapBinding.IsPushPermissionGranted();
Available Callbacks for Push Primer
Based on whether the notification permission is granted or denied, the CleverTap Unity Native SDK provides a callback with the permission status.
// returns the status of the push permission response after it's granted/denied
void CleverTapOnPushPermissionResponseCallback(string message) {
//Ensure to create the `CreateNotificationChannel` once notification permission is granted to register for receiving push notifications for Android 13+ devices.
Debug.Log("unity received push permission response: " + (!String.IsNullOrEmpty(message) ? message : "NULL"));
}
Updated 4 months ago