Unity SDK Quick Start Guide (Legacy)
Overview
This section shows how to install the CleverTap SDK, track your first user event, and see this information within the CleverTap dashboard in less than ten minutes. CleverTap provides Unity SDK that enables app developers to track, segment, and engage their users.
Install
To install the SDK:
- Import the
CleverTapUnityPlugin.unitypackage
into your Unity Project (Assets > Import Package > Custom Package) or manually copyPlugin/CleverTapUnity
,Plugin/PlayServicesResolver
andPlugin/Plugins/Android
(or copy the files inPlugin/Plugins/Android
to your existing Assets > Plugins > Android` directory) into the Assets directory of your Unity Project. - Create an empty game object (GameObject > Create Empty) and rename it as
CleverTapUnity
. AddAssets/CleverTapUnity/CleverTapUnity-Scripts/CleverTapUnity.cs
as a component of theCleverTapUnity GameObject
.
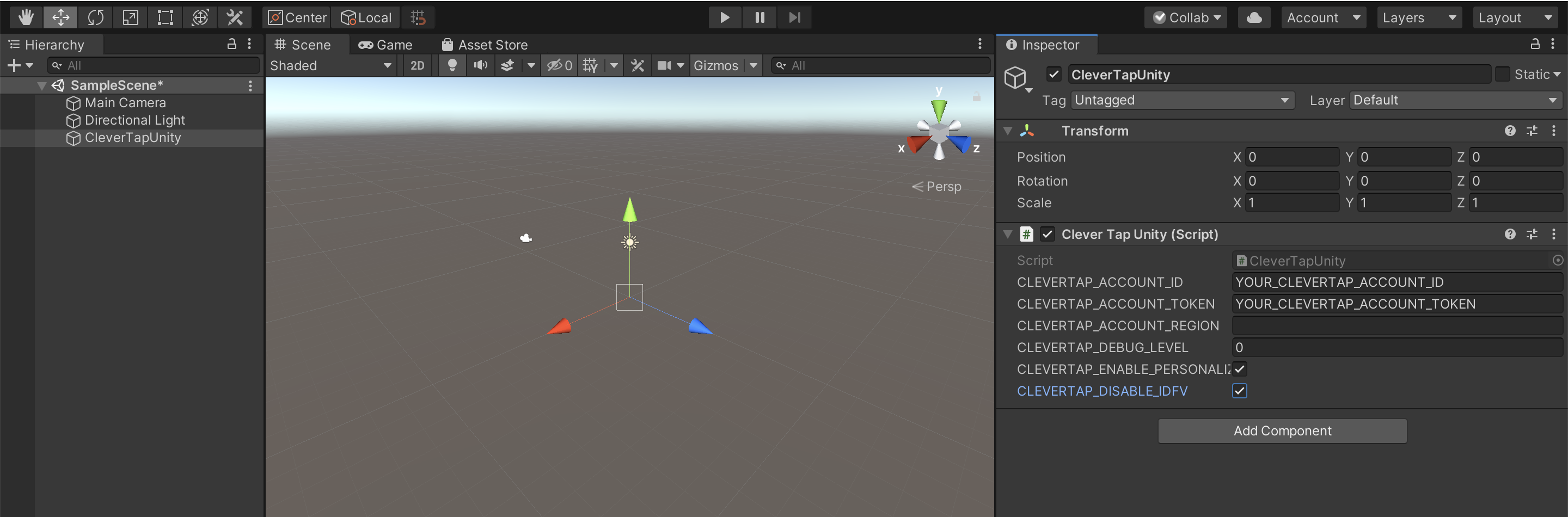
Create a Sample(CleverTapUnity) Game Object
- Select the
CleverTapUnity GameObject
that you created in the Hierarchy pane and add your CleverTap settings inside the Inspector window. You must include yourCleverTap Account ID
andCleverTap Account Token
from the Settings page.
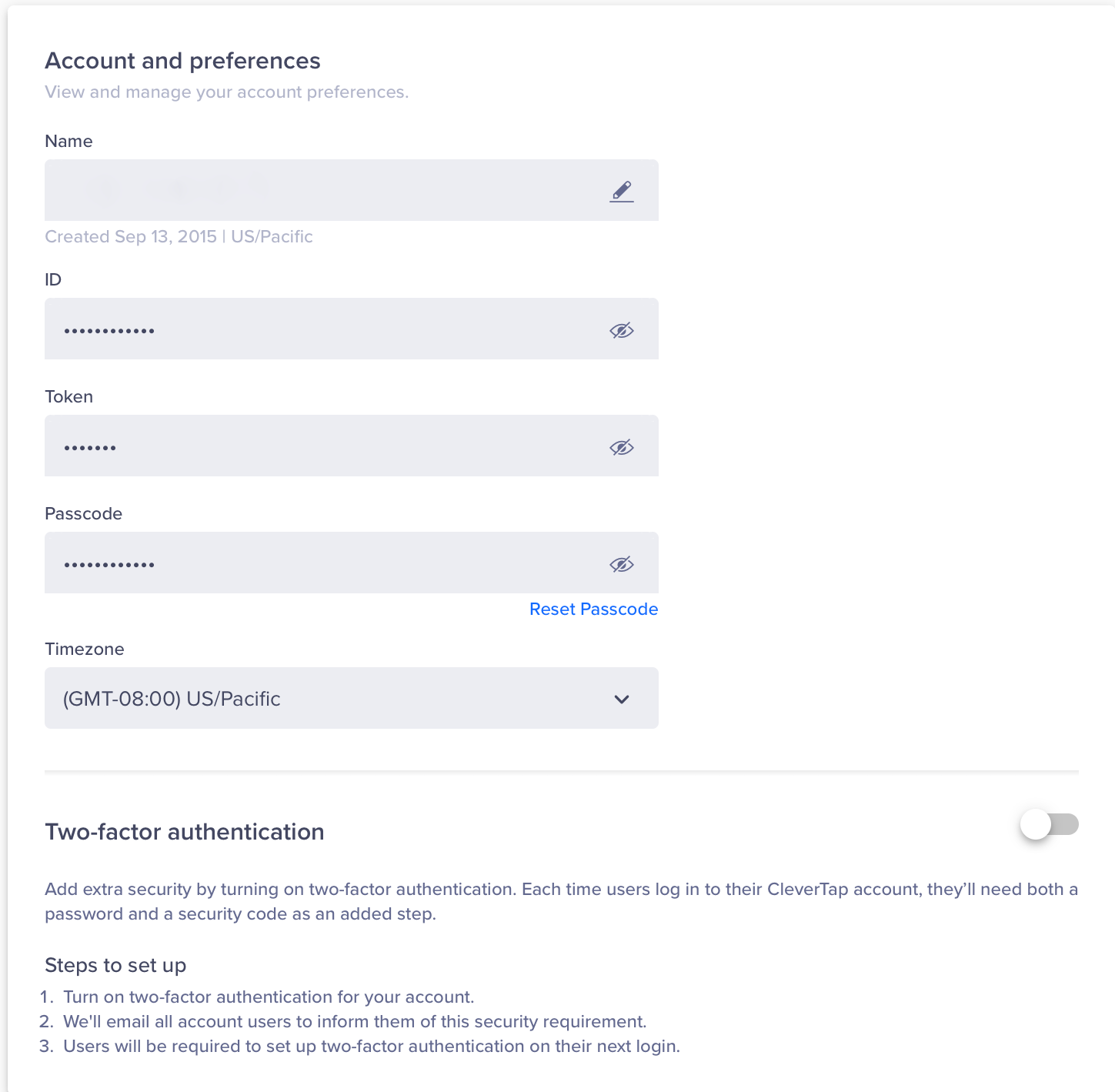
Add CleverTap Account and Preferences
- Edit
Assets/CleverTapUnity/CleverTapUnity-Scripts/CleverTapUnity.cs
to add your calls to the CleverTap SDK. See usage examples in example/CleverTapUnity.cs. For more information, refer to the Developer Guide.
IDFV Usage for CleverTap ID
Starting from CleverTap Unity SDK 2.1.2, you can optionally disable the usage of IDFV for CleverTap ID by selecting CLEVERTAP_DISABLE_IDFV. We recommend this for apps that send data from different iOS apps to a common CleverTap account. By default, the CLEVERTAP_DISABLE_IDFV checkbox is cleared.
Add Region Code
To know how to add region code in Unity SDK, refer to Region Codes.
iOS Specific Instructions
- To enable Push Notifications, ensure to add the Push Notifications capability to your Xcode project.
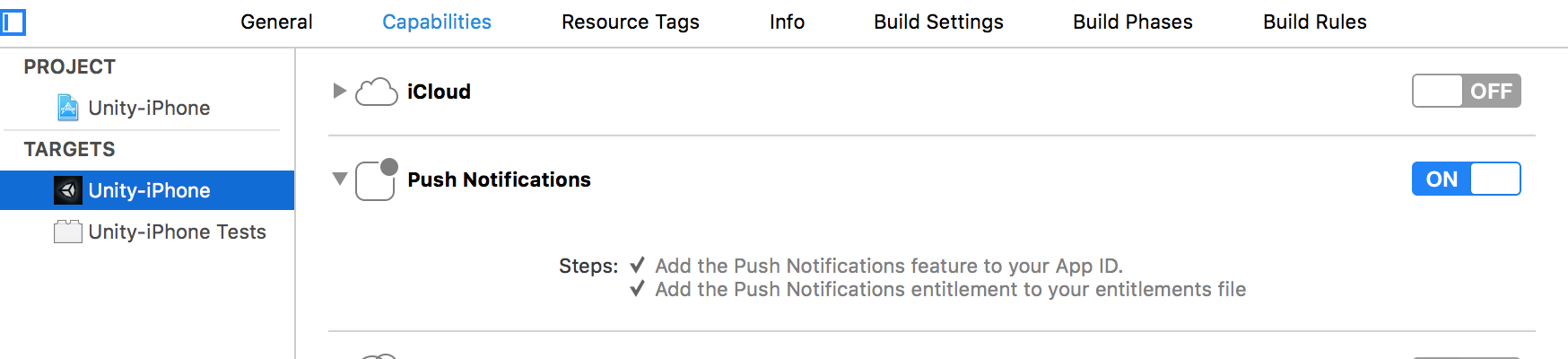
Enable Push Notifications
- Add a run script to your build phases. In Xcode, go to your Targets, under your appβs name, select Build Phases after embed frameworks, add a run script phase, and set it to use
/bin/sh
and the iOS script:
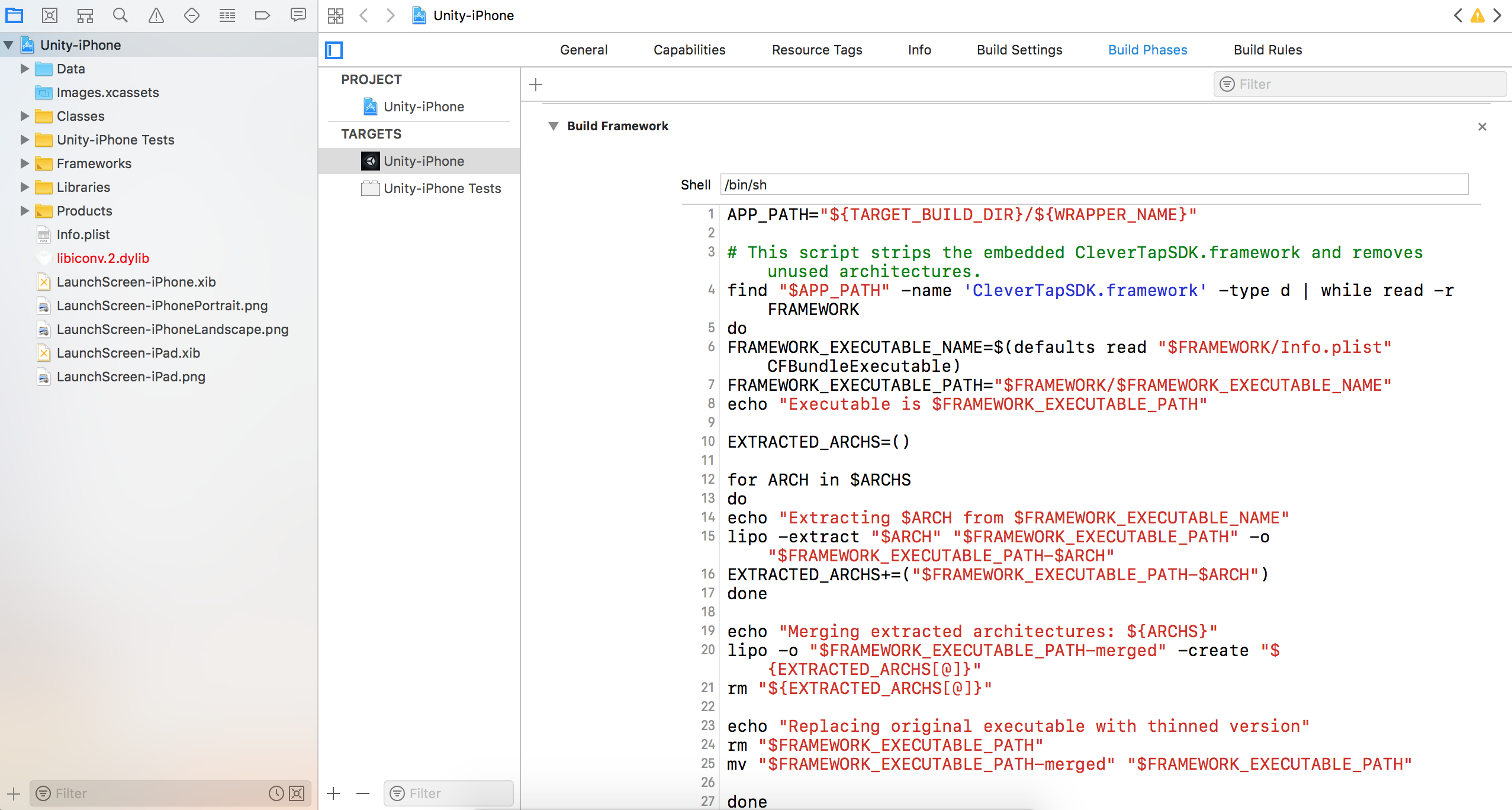
Add a Run Script
The script scans your built applicationβs Frameworks
folder and strips the unnecessary simulator architectures from the CleverTakSDK.framework
prior to archiving/submitting the app store.
- Build and run your iOS project.
Android Specific Instructions
Steps for Android X Dependencies
Perform these additional steps only if you are using Android X dependencies.
- Go to File > Build Settings > Android > Player Settings > Publishing Settings > Build.
- Ensure the
Custom Gradle Template
option is selected.- Go to Assets > Play Services Resolver > Android Resolver > Settings.
- Ensure that
Use Jetifier
andPatch mainTemplate.gradle
options are selected.
- To enable Push Notifications, add the Firebase Unity SDK to your app. For more information, refer to Firebase Unity Setup Docs.
Note
The Firebase Unity SDK may override your
AndroidManifest.xml
file. If that happens, revert to your original manifest file.
- Run Assets > Play Services Resolver > Android Resolver > Resolve Client Jars from the Unity menu bar to install the required google play services and android support library dependencies.
- Edit the
AndroidManifest.xml
file inAssets/Plugins/Android
to add your Bundle Identifier, FCM Sender ID, CleverTap Account Id, CleverTap Token, and Deep Link URL scheme (if applicable):
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="YOUR_BUNDLE_IDENTIFIER" android:versionName="1.0" android:versionCode="1" android:installLocation="preferExternal"> <supports-screens android:smallScreens="true" android:normalScreens="true" android:largeScreens="true" android:xlargeScreens="true" android:anyDensity="true" />
<meta-data
android:name="CLEVERTAP_ACCOUNT_ID"
android:value="Your CleverTap Account ID"/>
<meta-data
android:name="CLEVERTAP_TOKEN"
android:value="Your CleverTap Account Token"/>
<!-- Deep Links uncomment and replace YOUR_URL_SCHEME, if applicable, or remove if not supporting deep links-->
<!--
<intent-filter android:label="@string/app_name">
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="YOUR_URL_SCHEME" />
</intent-filter>
-->
- Add the following in the
AndroidManifest.xml
file:
<service
android:name="com.clevertap.android.sdk.pushnotification.fcm.FcmMessageListenerService">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>
- Add your
google-services.json
file to the project's Assets folder. - Build your app or Android project as usual.
Initialize CleverTap SDK
#if (UNITY_IPHONE)
CleverTapBinding.LaunchWithCredentials(CLEVERTAP_ACCOUNT_ID, CLEVERTAP_ACCOUNT_TOKEN);
#endif
#if (UNITY_ANDROID)
CleverTapBinding.Initialize(CLEVERTAP_ACCOUNT_ID, CLEVERTAP_ACCOUNT_TOKEN);
#endif
Track User Profiles
Create a User profile when the user logs in (On User Login):
Dictionary<string, string> newProps = new Dictionary<string, string>();
newProps.Add("Name", "Jack Montana"); // String
newProps.Add("Identity", "61026032"); // String
newProps.Add("Email", "[email protected]"); // Email address of the user
newProps.Add("Phone", "+14155551234"); // Phone (with the country code, starting with +)
newProps.Add("Gender", "M"); // Can be either M or F
CleverTapBinding.OnUserLogin(newProps);
Track User Events
Record an event without event data:
// record basic event with no properties
CleverTapBinding.RecordEvent("testEvent");
Record an event with event data:
// record event with properties
Dictionary<string, object> Props = new Dictionary<string, object>();
Props.Add("testKey", "testValue");
CleverTapBinding.RecordEvent("testEventWithProps", Props);
Updated about 2 months ago