CleverTap Huawei Push Integration
Learn how to integrate and send push notifications from Huawei.
Huawei uses its own push service to deliver notifications to their devices.
CleverTap can send push notifications powered by Huawei Cloud Push, an Android push notification delivery service. We send a push notification through both Huawei Cloud Push and Firebase Cloud Messaging push services for a greater chance of delivery success. If a message is delivered through one of these push services, the notification from the other cloud service is suppressed. This ensures that the user will only receive the push notification once.
Register as a Huawei Developer
The first step to accessing the Huawei cloud push is to register as a Huawei developer on the Huawei Website.
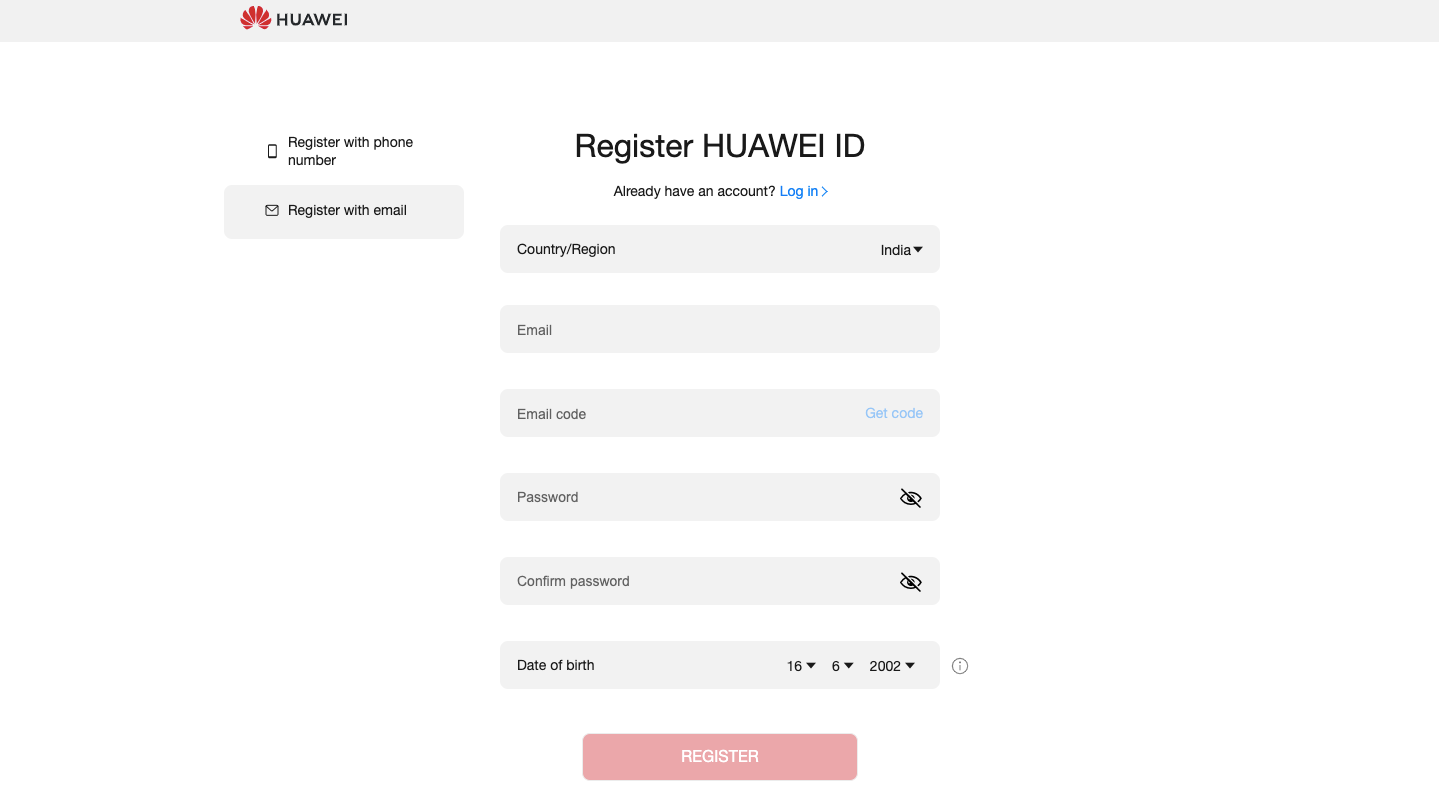
Register Huawei Developer
Enable the Push Service
In the Huawei console, enable the push kit. Enable other settings as mentioned by Huawei here
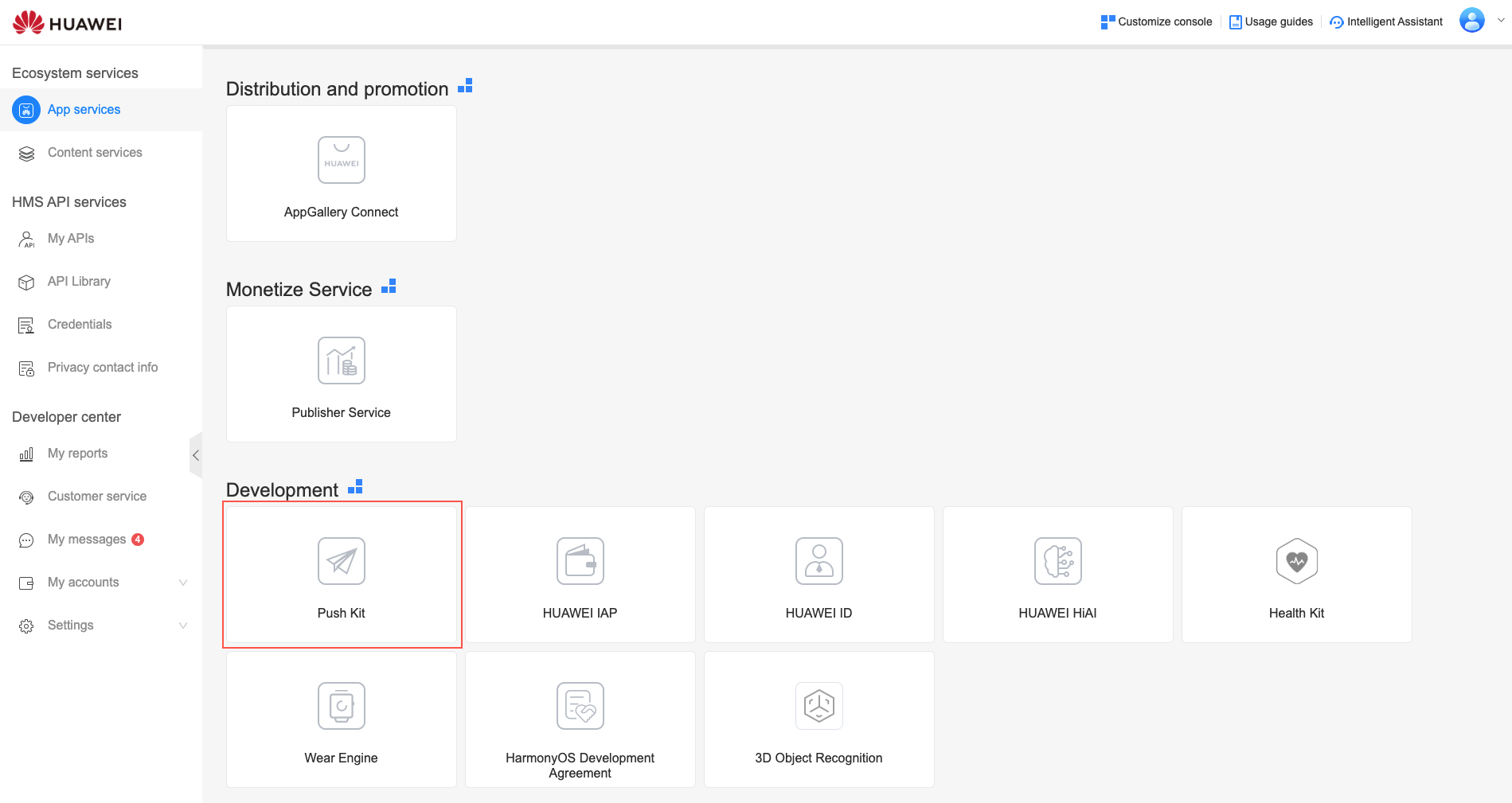
Enable the Push Service
Get the app's App ID/ App Secret
Once the App is created on your console, click on the App name to get your App ID/ App Secret. Among these, the AppID and AppKey are the client’s identity, used when the client SDK initializes; the AppSecret is authenticated for sending a message at the server-side.
Integrate Huawei HMS SDK
Huawei push notifications can be integrated in two ways, either use the CleverTap HMS SDK or integrate the Huawei HMS SDK manually.
Refer to the Huawei checklist for integration preparation.
Download the agconnect-services.json
file from the Huawei Console. Move the downloaded agconnect-services.json
file to the app directory of your Android Studio project.
Method 1 - Configure Manifest and Gradle files with CleverTap HMS SDK
It is easier to configure the Huawei push with CleverTap HMS SDK.
Step 1
Configure the root-level build.gradle file.
buildscript {
repositories {
// HUAWEI ADD THIS
maven {url 'http://developer.huawei.com/repo/'}
}
dependencies {
classpath "com.android.tools.build:gradle:7.3.0"
// FOR HUAWEI ADD THIS
classpath "com.huawei.agconnect:agcp:1.9.0.300" //check the version of the latest compatible library here : https://github.com/CleverTap/clevertap-android-sdk/blob/master/docs/CTHUAWEIPUSH.md
}
}
allprojects {
repositories {
// HUAWEI ADD THIS
maven {url 'http://developer.huawei.com/repo/'}
}
}
Step 2
Configure the build.gradle at the app-level.
implementation "com.clevertap.android:clevertap-hms-sdk:1.3.3"
implementation "com.huawei.hms:push:6.11.0.300"
//At the bottom of the file add this
apply plugin: 'com.huawei.agconnect
Method 2 - Configure Manifest and Gradle files manually
Set up your app per the configuration provided by Huawei
Implement a Service class that extends the HmsMessageService
class
HmsMessageService
classIn order to receive messages, you must implement a Service inherited from HmsMessageService and override all required methods in it: onNewToken
, onMessageReceived
, onMessageSent
and onSendError
and then register your Service in the AndroidManifest.xml
file. Method onMessageReceived
is used to receive messages sent by CleverTap.
Pass token to CleverTap
In your Service class that extends the HmsMessageService
class, inside the onNewToken
method, pass the token to CleverTap using the following code:
public void onNewToken(@Nullable String token, @Nullable Bundle bundle) {
super.onNewToken(token, bundle);
if(token!=null){
cleverTapAPIInstance.pushHuaweiRegistrationId(token, true);
}
}
override fun onNewToken(token: String?, bundle: Bundle?) {
super.onNewToken(token, bundle)
if (token != null) {
cleverTapAPIInstance.pushHuaweiRegistrationId(token, true)
}
}
Receive Push Notifications from CleverTap
In the onMessageReceived
method, write the following code for CleverTap to render Push Notification and raise Notification Viewed event.
@Override
public void onMessageReceived(RemoteMessage message) {
try {
String ctData = message.getData();
Bundle extras = Utils.stringToBundle(ctData);
CleverTapAPI.createNotification(getApplicationContext(),extras);
} catch (JSONException e) {
e.printStackTrace();
}
}
fun onMessageReceived(message:RemoteMessage) {
try
{
val ctData = message.getData()
val extras = Utils.stringToBundle(ctData)
CleverTapAPI.createNotification(getApplicationContext(), extras)
}
catch (e:JSONException) {
e.printStackTrace()
}
}
From CleverTap HMS SDK v1.2.0, you can replace the above code with the following snippet:
@Override
public void onMessageReceived(RemoteMessage message) {
CTHmsMessageHandler().createNotification(getApplicationContext(),message);
}
fun onMessageReceived(message:RemoteMessage) {
CTHmsMessageHandler().createNotification(applicationContext,message)
}
Note
CleverTap Android SDK v5.1.0 onwards, the following API now runs on the caller's thread. Make sure to call it on background thread in
onMessageReceive()
of messaging service:
CTHmsMessageHandler().createNotification(getApplicationContext(), message)
CleverTapAPI.createNotification(getApplicationContext(),extras);
Update App ID/App Secret in Settings Dashboard
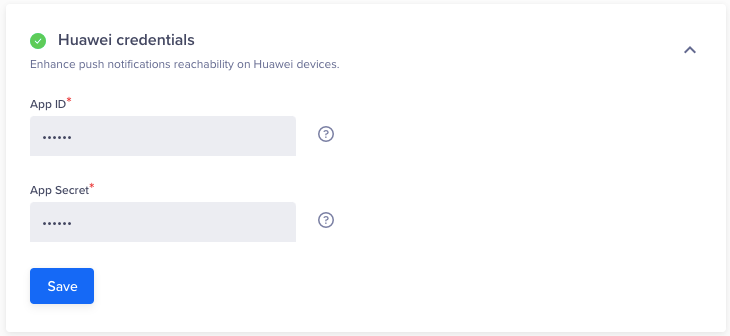
Update App ID/Secret in the Settings Dashboard
Updated 5 months ago