iOS Rich Push Notifications
Overview
iOS 10 has introduced rich push notifications, which provides the capability to add image, video, audio, or GIF attachments to your push notifications. These are great for short interactions that do not require the full app experience, and represent the growing importance of notifications to an app's user experience.
Rich push notifications are enabled via Notification Service extension and Notification Content extension. These are separate and distinct binary embedded in your app bundle. Prior to displaying a new push notification, the system will call your notification service extension to modify the payload as well as add media attachments to be displayed. Notification content extension is used to display a custom interface for your appβs notifications
Enable Rich Push Notifications
To enable rich push notifications:
- Enable push notifications for your app.
- Create a notification service extension in your project, in Xcode, select File > New > Target.
- Select the Notification Service Extension template.
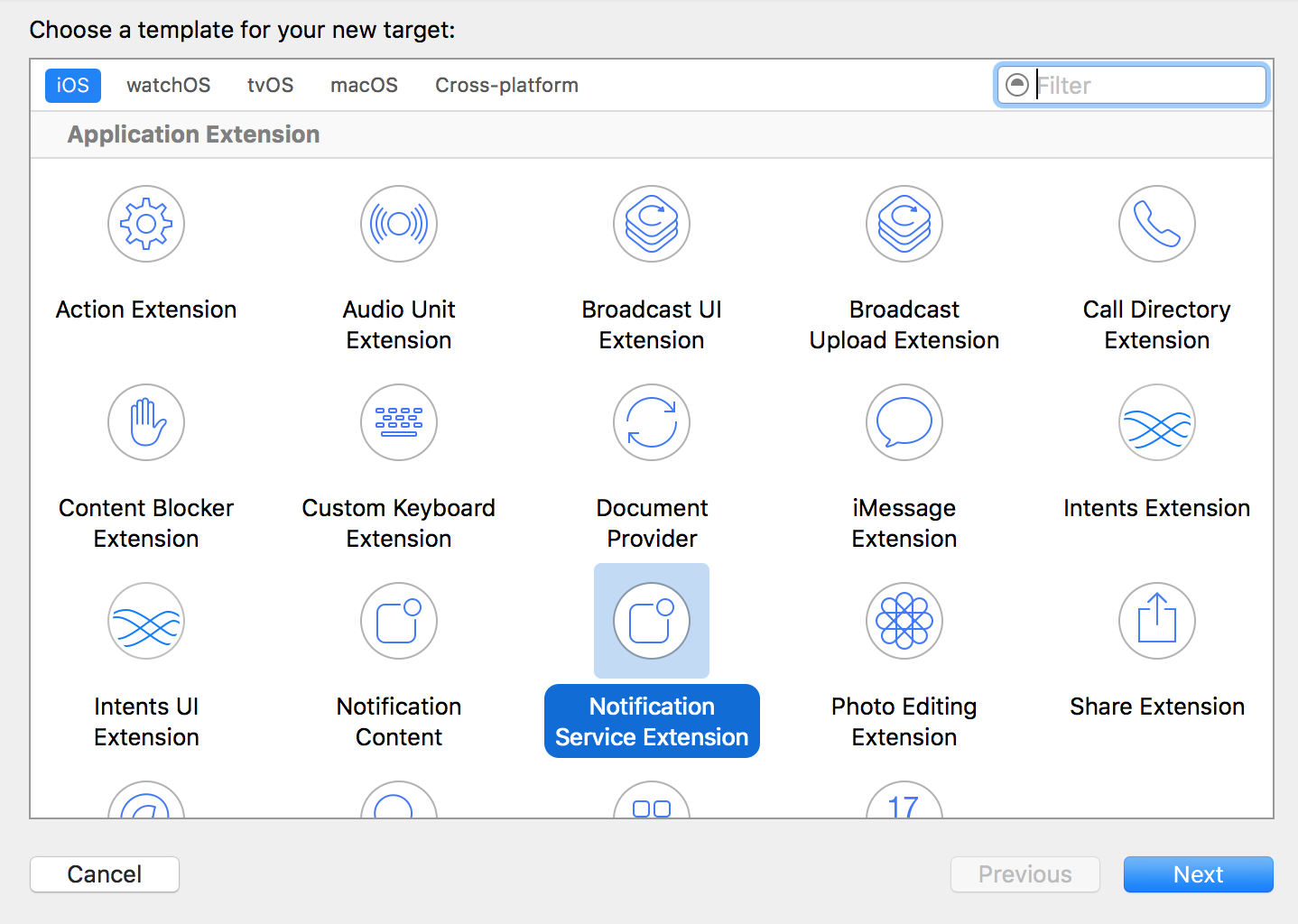
Notification Service Extension Template
- Install CTNotificationService in your Notification Service Extension. You can install with via CocoaPods(recommended), Carthage and manually.
Your Podfile should look something like this:
source 'https://github.com/CocoaPods/Specs.git'
platform :ios, '10.0'use_frameworks!
target 'YOUR_NOTIFICATION_SERVICE_TARGET_NAME' do
pod 'CTNotificationService'
end
Then run pod install
Configure your Notification Service Extension to use the CTNotificationServiceExtension class
By default CTNotificatonServiceExtension will look for the push payload key ct_mediaUrl with a value representing the url to your media file and the key ct_mediaType with a value of the type of media (image, video, audio or gif).
If you are happy with the default key names, you can simply insert CTNotificationServiceExtension in place of your extension class name as the value for the NSExtension -> NSExtensionPrincipalClass entry in your Notfication Service Extension target Info.plist.
Alternatively, you can leave the NSExtensionPrincipalClass entry unchanged and instead have your NotificationService class extend the CTNotificationServiceExtension class. You can then also override the defaults to your chosen key names if you wish. In that case, only override didReceive request: contentHandler: as shown in the example.
If you plan on downloading non-SSL urls please be sure to enable App Transport Security Settings -> Allow Arbitrary Loads -> true in your plist.
Configure your APNS payload
Then, when sending notifications via APNS:
Required Key
Include the mutable-content flag in your payload aps entry (this key must be present in the aps payload or the system will not call your app extension)
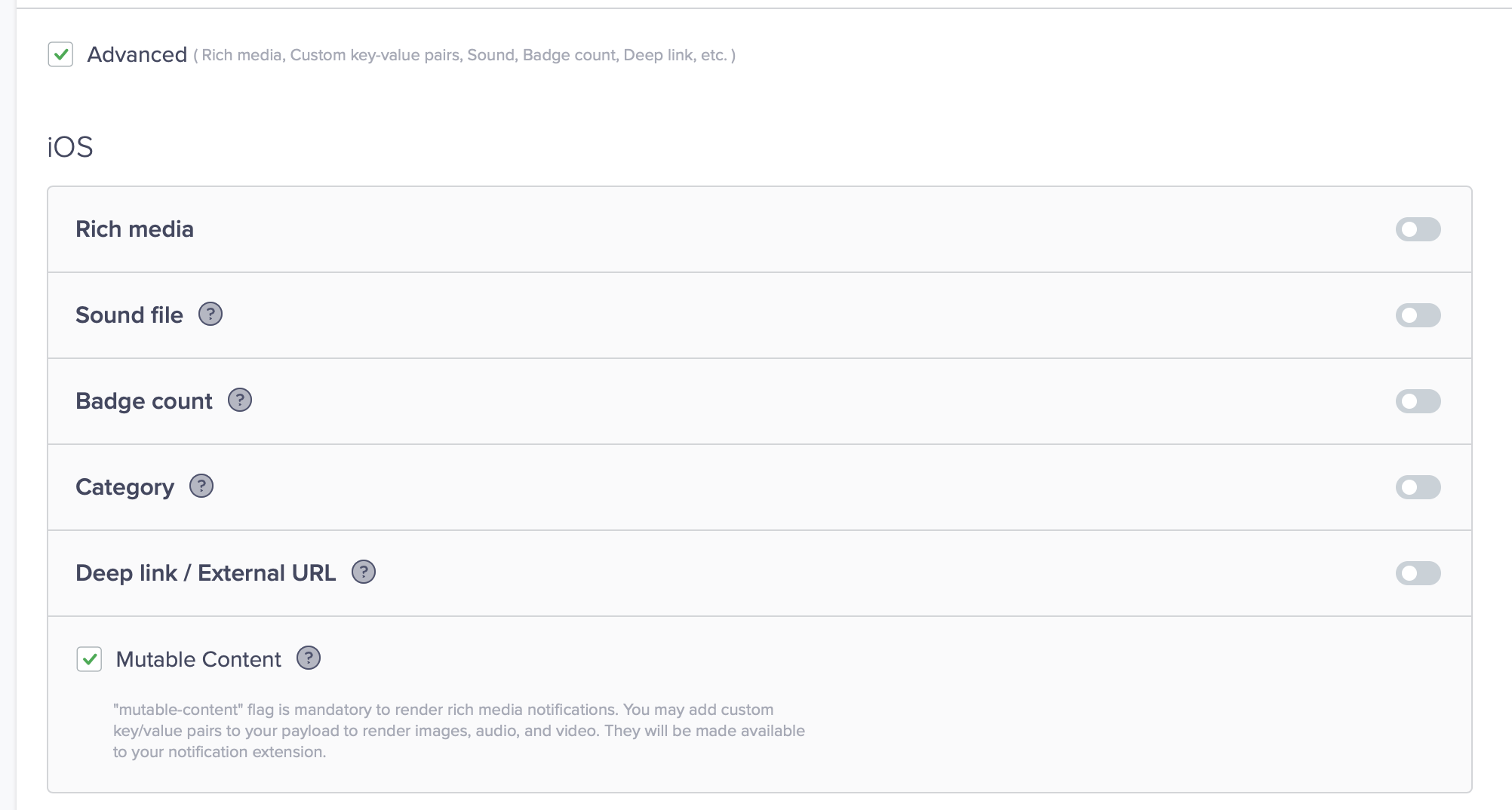
Configure APNS Payload
Add the ct_mediaUrl and ct_mediaType key-values (or your custom key-values) to the payload, outside of the aps entry.
{
"aps": {
"alert": {
"body": "test message",
"title": "test title",
},
"mutable-content": 1,
},
"ct_mediaType": "gif",
"ct_mediaUrl": "https://www.wired.com/images_blogs/design/2013/09/davey1_1.gif",
...
}
Your application is now configured to show push notifications with single media. To see Image captions, sub-captions and carousel media, you need to add CTNotificationContent in your Notification Content Extension. You can install with via CocoaPods(recommended), Carthage and manually.
Your Podfile should look something like this:
source 'https://github.com/CocoaPods/Specs.git'
platform :ios, '10.0'use_frameworks!
target 'YOUR_NOTIFICATION_CONTENT_TARGET_NAME' do
pod 'CTNotificationContent'
end
Then run pod install
- Create a Notification Content Extension in your project. To do that in your Xcode project, select File -> New -> Target and choose the Notification Content Extension template.
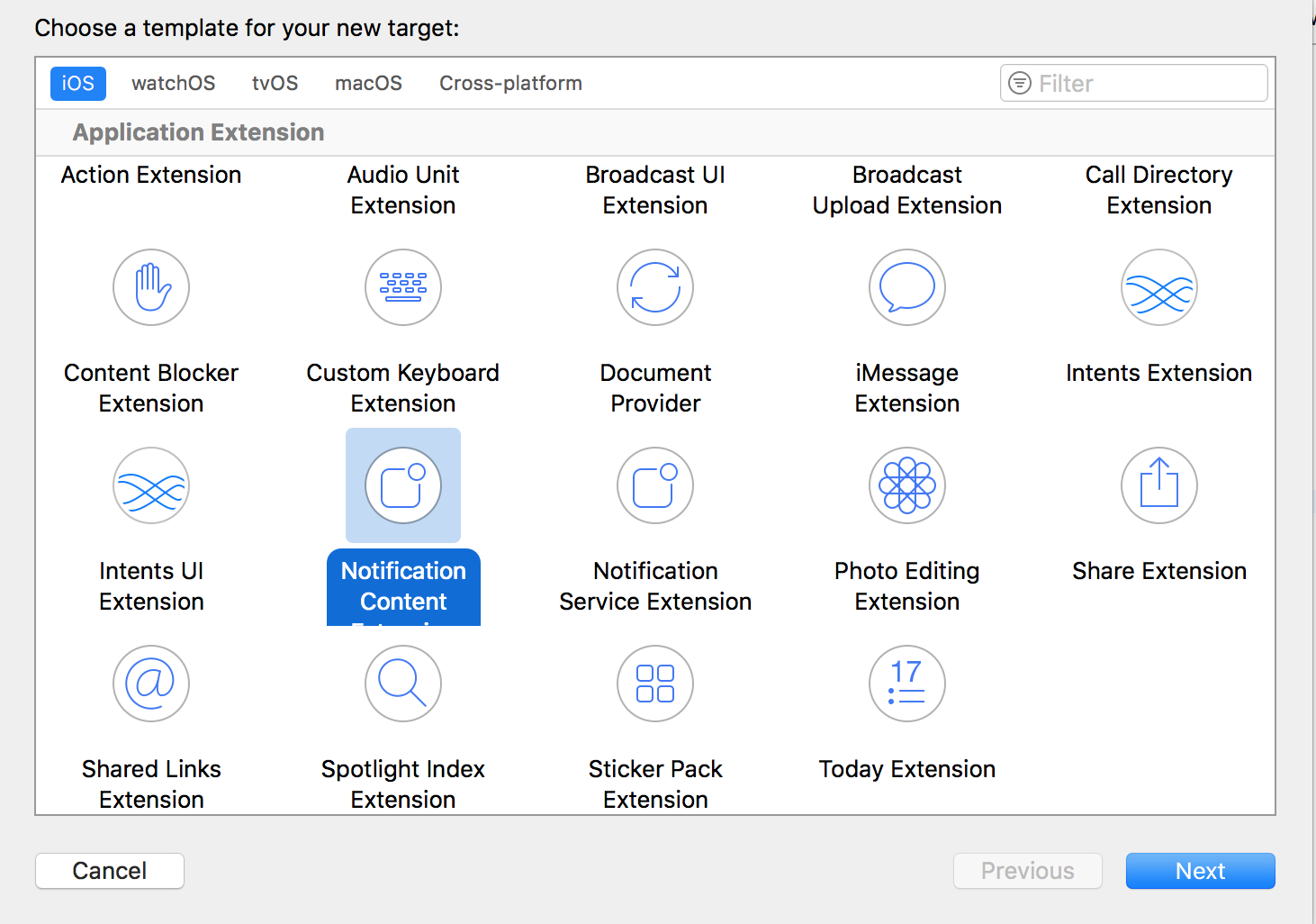
Notification Content Extension Template
Configure your Notification Content Extension to use the CTNotificationViewController class
Change the superclass of your NotificationViewController to CTNotificationViewController. You should not implement any of the UNNotificationContentExtension protocol methods in your NotificationViewController class, those will be handled by CTNotificationViewController. See Objective-C example here and Swift example here.
Edit the Maininterface.storyboard in your NotificationContent target to a plain UIView.
In your AppDelegate, register the Notification category and actions:
// register category with actions
let action1 = UNNotificationAction(identifier: "action_1", title: "Back", options: [])
let action2 = UNNotificationAction(identifier: "action_2", title: "Next", options: [])
let action3 = UNNotificationAction(identifier: "action_3", title: "View In App", options: [])
let category = UNNotificationCategory(identifier: "CTNotification", actions: [action1, action2, action3], intentIdentifiers: [], options: [])
UNUserNotificationCenter.current().setNotificationCategories([category])
UNUserNotificationCenter *center = [UNUserNotificationCenter currentNotificationCenter];
UNNotificationAction *action1 = [UNNotificationAction actionWithIdentifier:@"action_1" title:@"Back" options:UNNotificationActionOptionNone];
UNNotificationAction *action2 = [UNNotificationAction actionWithIdentifier:@"action_2" title:@"Next" options:UNNotificationActionOptionNone];
UNNotificationAction *action3 = [UNNotificationAction actionWithIdentifier:@"action_3" title:@"View In App" options:UNNotificationActionOptionNone];
UNNotificationCategory *cat = [UNNotificationCategory categoryWithIdentifier:@"CTNotification" actions:@[action1, action2, action3] intentIdentifiers:@[] options:UNNotificationCategoryOptionNone];
[center setNotificationCategories:[NSSet setWithObjects:cat, nil]];
Then configure your Notification Content target Info.plist to reflect the category identifier you registered: NSExtension -> NSExtensionAttributes -> UNNotificationExtensionCategory. In addition, set the UNNotificationExtensionInitialContentSizeRatio -> 0.1 and UNNotificationExtensionDefaultContentHidden -> true.
Also, If you plan on downloading non-SSL urls please be sure to enable App Transport Security Settings -> Allow Arbitrary Loads -> true in your plist. See plist example here.
Send Rich Push Notifications from dashboard
When using the CleverTap Dashboard to send rich push notifications, create a Push Notification campaign on CleverTap and follow the steps below -
- On the "WHAT" section pass the desired required values in the "title" and "message" fields (NOTE: These are iOS alert title and body).
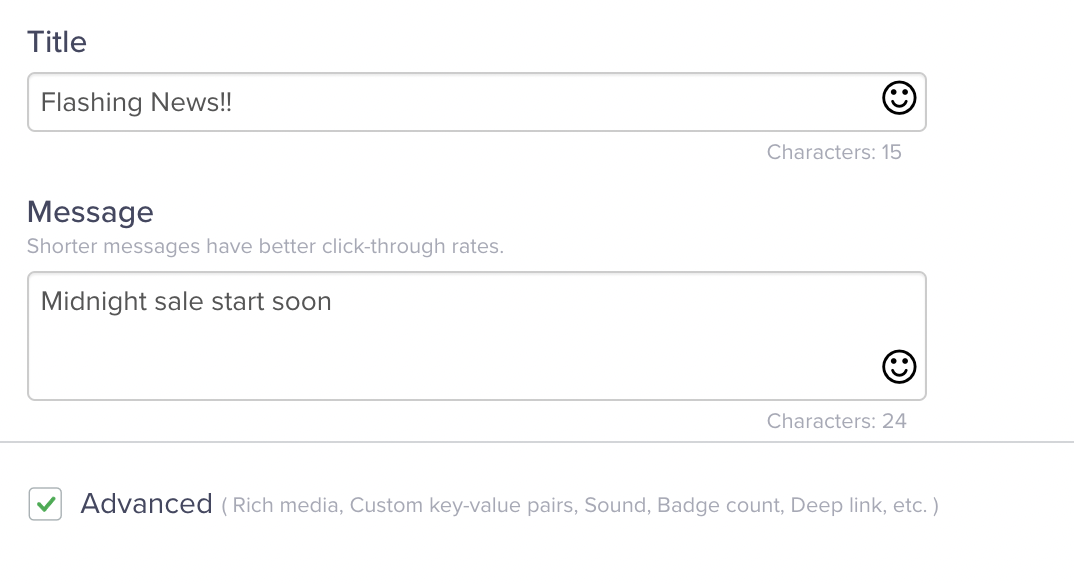
Configure What Section
- Click on "Advanced" and then click on "Rich Media" and select Single or Carousel template.
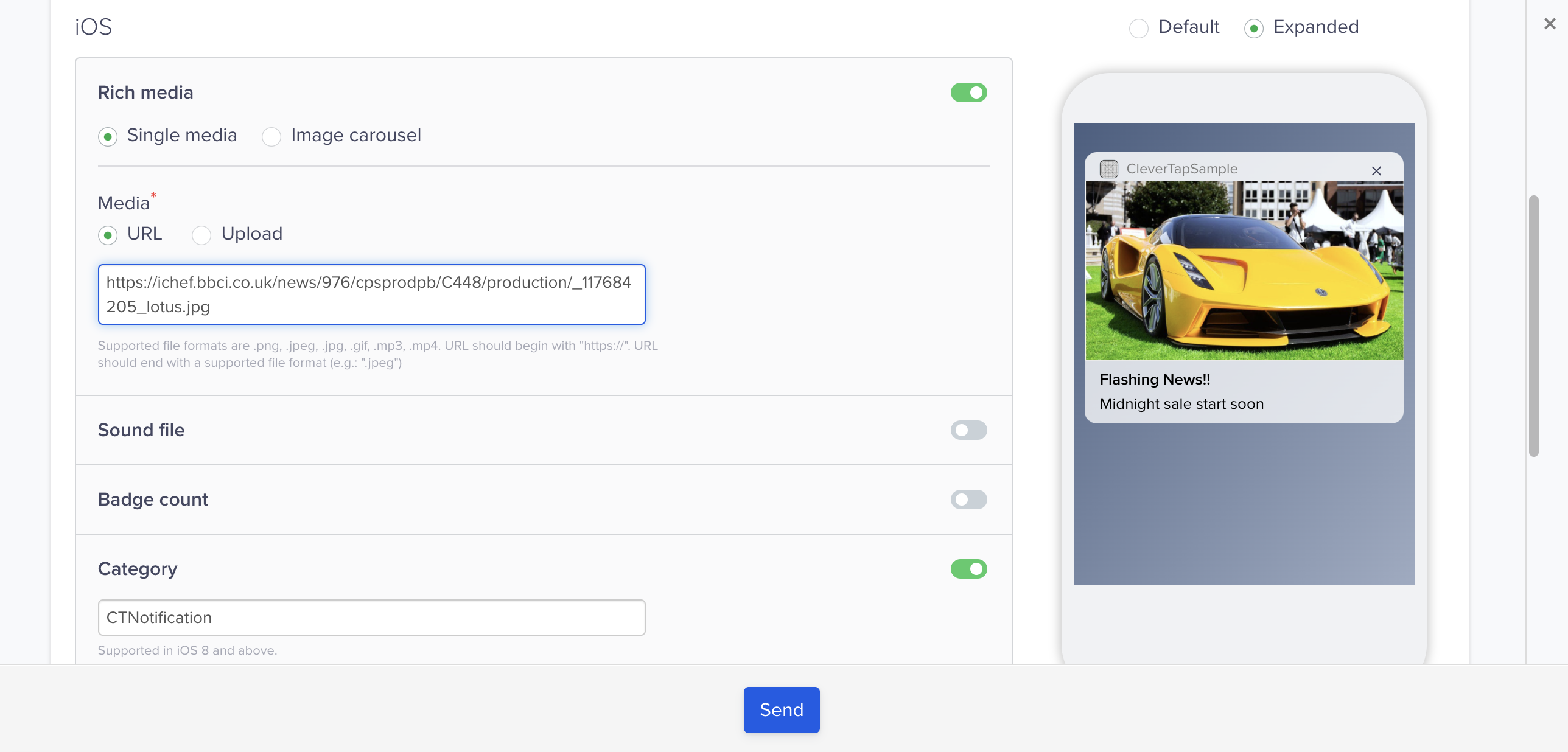
Configure Rich Push Notification
- Add your custom key-value pair(s). For adding custom key-value pair, add the template Keys individually or into one JSON object and use the
pt_json
key to fill in the values. Refer to this page for more template keys.
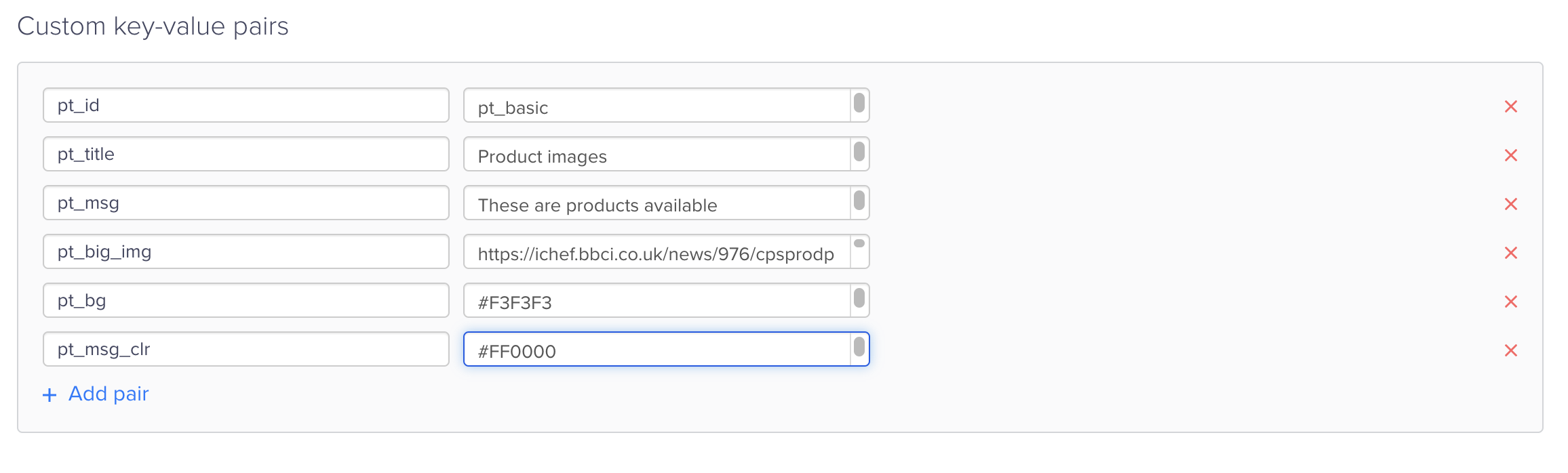
Add Custom Key-Value Pair

Update Custom Key-Value Pair
When using the CleverTap Server API to send push: include "mutable-content": "true" in the platform_specific: iOS section of the request payload. Schedule the push notification.
Rich Push Templates supported by CleverTap:
Rich Media
- Single Media
Single media is for basic view with single image.
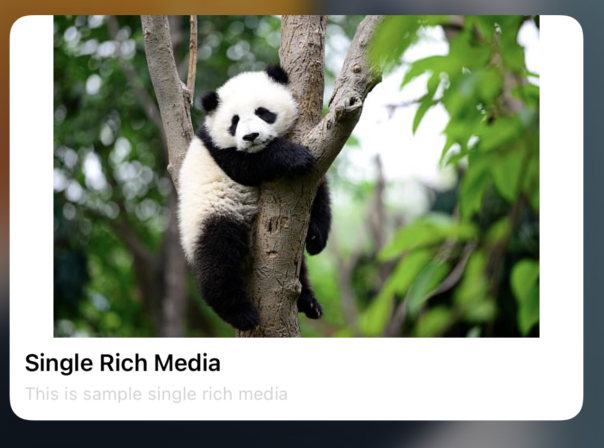
Single Rich Media
- Content Slider
Content Slider is for image slideshow view where user can add multiple images with different captions, sub-captions, and actions.
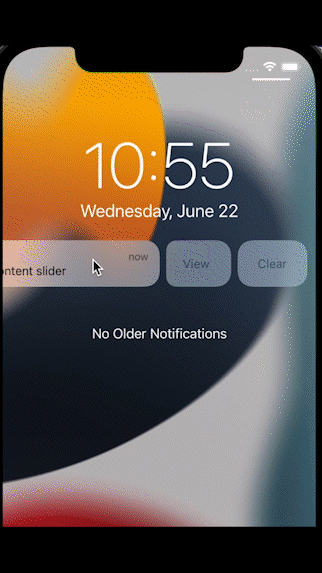
Content Slider
Custom key-value pair
- Basic Template
Basic Template is the basic push notification received on apps where user can also update text colour, background colour.
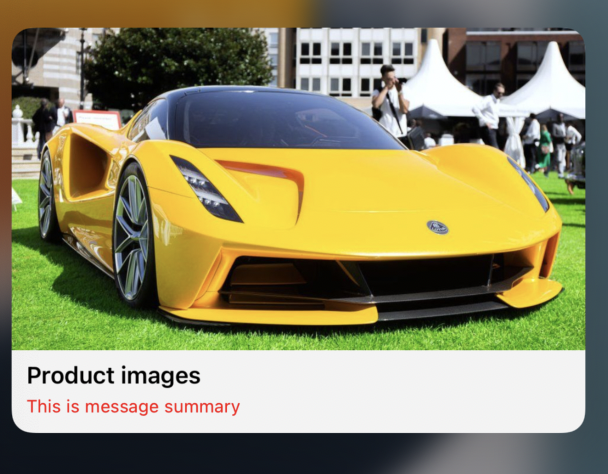
Basic Template
- Auto Carousel Template
Auto carousel is an automatic revolving carousel push notification where user can also update text colour, background colour.
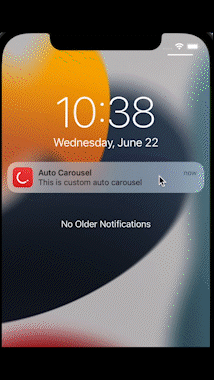
Auto Carousel Template
- Manual Carousel Template
This is the manual version of the carousel. The user can navigate to the next/previous image by clicking on the Next/Back buttons.
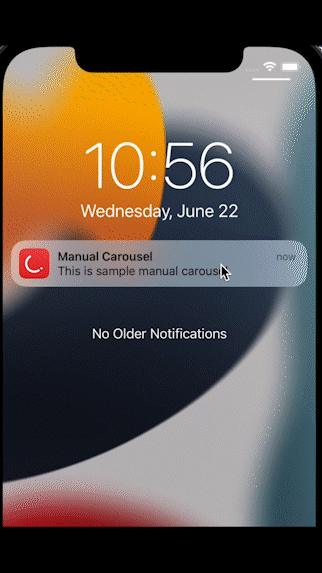
Manual Carousel Template
- Timer Template
This template features a live countdown timer. You can even choose to show different title, message, and background image after the timer expires.
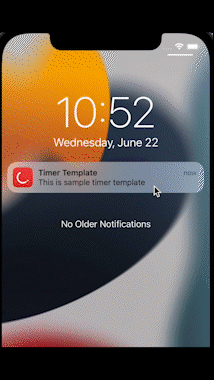
Timer Template
Note
If any image can't be downloaded, the template falls back to basic template with caption and sub caption only.
Examples
Below are some project examples:
Updated about 1 year ago