Android In-App
Learn how to send and control in-app notifications.
Overview
CleverTap provides the capability to send in-app messages with images, GIFs, video, and audio. You can also do A/B testing on your in-apps.
Note
Ensure your activities extend FragmentActivity.
Exclude in-app from Android Activity
If your application has a splash screen (for example, logo screen or loading page) displayed on app launch, then the in-app triggered on App Launch would be attempted to be displayed on this screen. As soon as this screen is dismissed, the in-app will be dismissed, too.
In these types of cases, the splash screen must be excluded. Mention the Activities on which you do not want in-app notifications to show in the android:value of the metadata tag in the AndroidManifest.xml file.
<meta-data
android:name="CLEVERTAP_INAPP_EXCLUDE"
android:value="YourSplashActivity1, YourSplashActivity2" />
Control In-App Notifications
With CleverTap Android SDK 4.2.0 and above, you can control the rendering of in-app notifications by calling the following methods:
Suspend
Suspends and saves in-app notifications until resumeInAppNotifications
method is called for the current session.
CleverTapSingleton.getDefaultInstance(ctx).suspendInAppNotifications();
CleverTapAPI.getDefaultInstance(ctx)!!.suspendInAppNotifications()
Discard
Suspends the display of in-app Notifications and discards the display of any new in-app notification. It also discards in-app notifications until resumeInAppNotifications
method is called for the current session.
CleverTapSingleton.getDefaultInstance(ctx).discardInAppNotifications();
CleverTapAPI.getDefaultInstance(ctx)!!.discardInAppNotifications()
Resume
The resumeInAppNotifications
method resumes displaying in-app notifications.
If you call this method after the discardInAppNotifications()
method, it resumes the in-app notifications for events raised after the call is performed.
However, if you call the resumeInAppNotifications
method after the suspendInAppNotifications()
method, then it displays all queued in-app notifications and also resumes in-app notifications for events raised after the call is performed.
CleverTapSingleton.getDefaultInstance(ctx).resumeInAppNotifications();
CleverTapAPI.getDefaultInstance(ctx)!!.resumeInAppNotifications()
Javascript Support in In-App Notifications
Android SDK v3.5.0 and above supports embedding JavaScript code inside custom in-apps. To ensure your JavaScript code works on the app while creating the in-app campaign, select the checkbox for Enabling JavaScript during the in-app campaign creation.
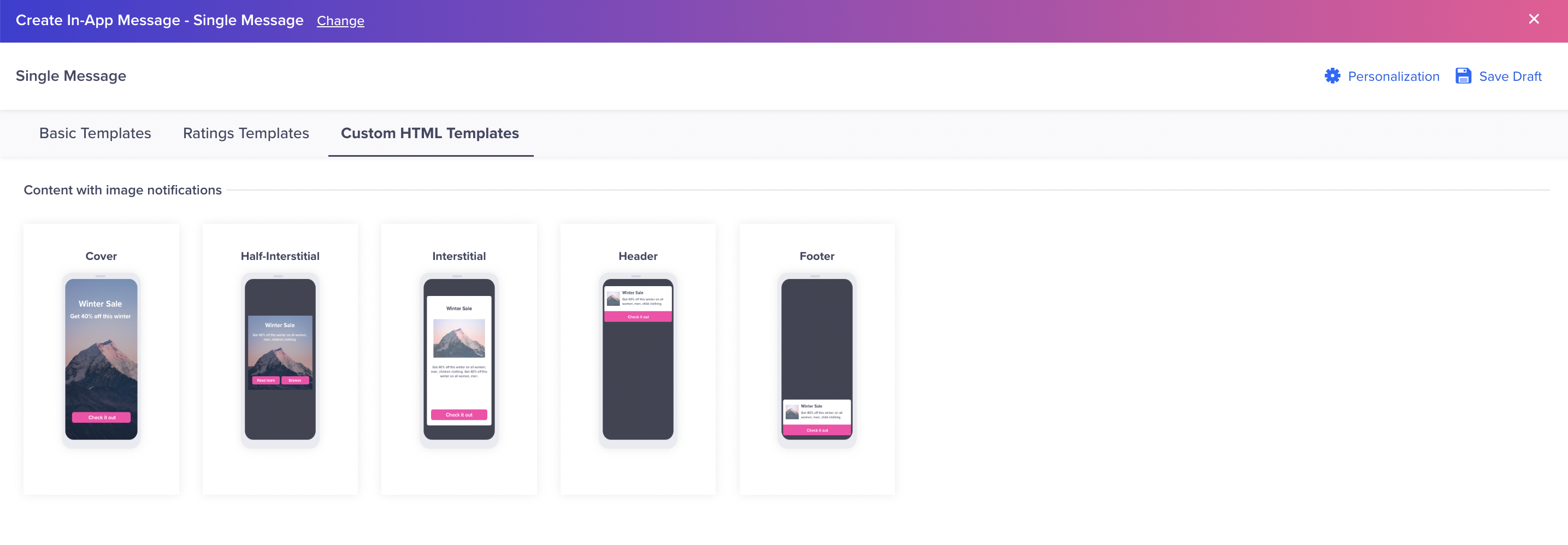
Custom HTML Template in In-App Notifications
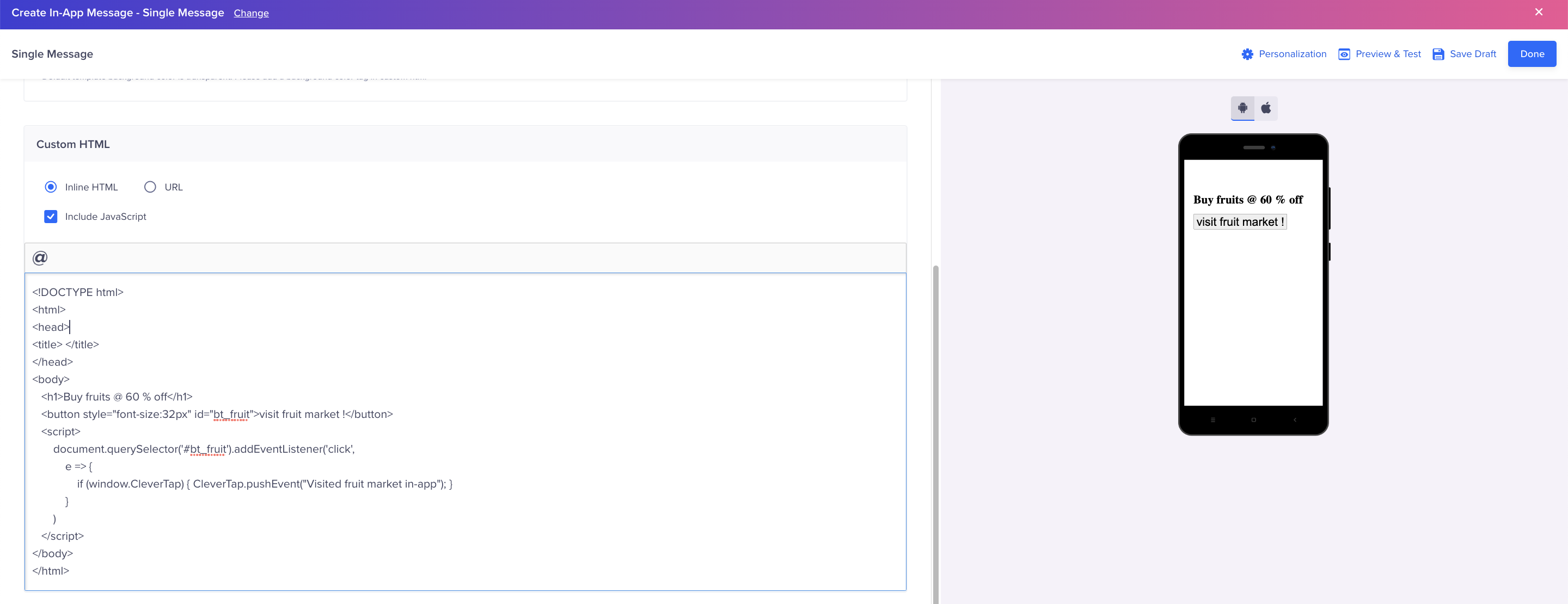
Include JavaScript in In-App Notifications
Consider the following methods explained with examples:
//Recording a User Event called Product Viewed in JS enabled custom In-Apps
if (window.CleverTap) {
// Call Android interface
CleverTap.pushEvent("Product Viewed");
}
//Recording a User Event called Product Viewed with properties, in JS enabled custom In-Apps
var props = {foo: 'xyz', lang: 'French'};
if (window.CleverTap) {
// Call Android interface
CleverTap.pushEvent("Product Viewed",JSON.stringify(props));
}
//Updating profile properties of the User in JS enabled custom In-Apps
var props = {foo: 'xyz', lang: 'French'};
if (window.CleverTap) {
// Call Android interface
CleverTap.pushProfile(JSON.stringify(props));
}
//Adding user property for the User in JS enabled custom In-Apps
if (window.CleverTap) {
// Call Android interface
CleverTap.addMultiValueForKey('membership', 'gold');
}
//Adding multiple user properties for the User in JS enabled custom In-Apps
var cars = ['Saab', 'Volvo', 'BMW', 'Kia'];
if (window.CleverTap) {
// Call Android interface
CleverTap.addMultiValuesForKey('Cars', JSON.stringify(cars));
}
//removing a user property for a specific key in JS enabled custom In-Apps
if (window.CleverTap) {
// Call Android interface
CleverTap.removeMultiValueForKey(‘Cars’, 'Saab');
}
//Removing multiple user properties for a specific key in JS enabled custom In-Apps
var cars = ['BMW', 'Kia'];
if (window.CleverTap) {
// Call Android interface
CleverTap.removeMultiValuesForKey('Cars', JSON.stringify(cars));
}
//Removing a user property by specifying a key in JS enabled custom In-Apps
if (window.CleverTap) {
// Call Android interface
CleverTap.removeValueForKey('Cars');
}
//Setting a user property by specifying the key in JS enabled custom In-Apps
var values = ['Mercedes','Bentley']
if (window.CleverTap) {
// Call Android interface
CleverTap.setMultiValueForKey('Cars', JSON.stringify(values));
}
// Increment user property value by specifying the key in JS enabled custom In-Apps
if (window.CleverTap) {
// Call Android interface
CleverTap.incrementValue('Cars',2);
}
// Decrement user property value by specifying the key in JS enabled custom In-Apps
if (window.CleverTap) {
// Call Android interface
CleverTap.decrementValue('Cars',2)
}
//Calling onUserLogin
var props = {Identity: '12434', Email: '[email protected]'};
if (window.CleverTap) {
// Call Android interface
CleverTap.onUserLogin(JSON.stringify(props));
}
In-App Notification Button onClick Callbacks
Android SDK v3.6.1 and above supports callback on the click of in-app notification buttons by returning a map of key-value pairs. To use this, make sure your activity implements the InAppNotificationButtonListener
and override the following method:
@Override
public void onInAppButtonClick(HashMap<String, String> hashMap) {
if(hashMap != null){
//Read the values
}
}
override fun onInAppButtonClick(hashMap: HashMap<String, String>?) {
// Read the values
}
Set the InAppNotificationButtonListener
using the following code:
cleverTapInstance.setInAppNotificationButtonListener(this);
cleverTapDefaultInstance?.apply {
setInAppNotificationButtonListener(this@YourAndroidActivity)
}
Also please note that you must define custom key value pairs to receive this callback.
Android Push Primer
A Push Primer can explain to your users the need for push notifications and helps improve your engagement rates. It is an InApp notification that shows your users the details of message types they can expect before requesting notification permission. It helps with the following:
- Educates your users about the purpose of requesting this permission before invoking a system dialog that asks the user to allow/deny this permission.
- Acts as a precursor to the hard system dialog and thus allows you to ask for permission multiple times if previously denied without making your users search the device settings.
For more information on push primer, refer to Push Notification Permission.
Push Primer using Half-Interstitial InApp
This template can send a customized push primer notification, such as displaying an image and modifying the text, button, and background color.
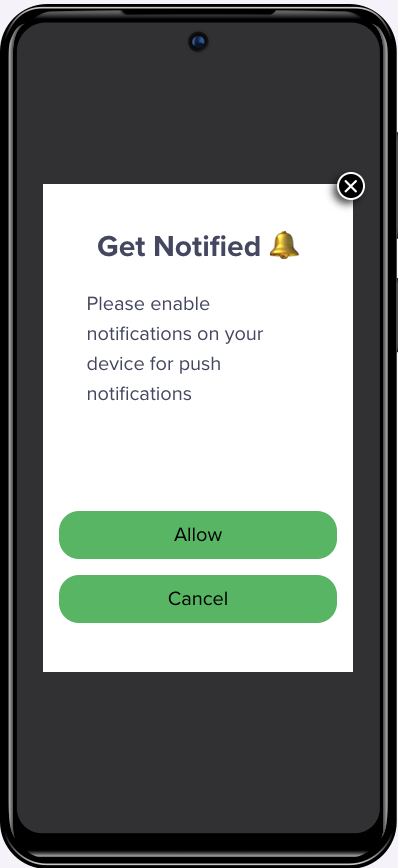
Push Primer Using Half-Interstitial InApp
Use the following to create your template:
JSONObject jsonObject = CTLocalInApp.builder()
.setInAppType(CTLocalInApp.InAppType.HALF_INTERSTITIAL)
.setTitleText("Get Notified")
.setMessageText("Please enable notifications on your device to use Push Notifications.")
.followDeviceOrientation(true)
.setPositiveBtnText("Allow")
.setNegativeBtnText("Cancel")
.setBackgroundColor(Constants.WHITE)
.setBtnBorderColor(Constants.BLUE)
.setTitleTextColor(Constants.BLUE)
.setMessageTextColor(Constants.BLACK)
.setBtnTextColor(Constants.WHITE)
.setImageUrl("https://icons.iconarchive.com/icons/treetog/junior/64/camera-icon.png")
.setBtnBackgroundColor(Constants.BLUE)
.build();
cleverTapAPI.promptPushPrimer(jsonObject);
val builder = CTLocalInApp.builder()
.setInAppType(CTLocalInApp.InAppType.HALF_INTERSTITIAL)
.setTitleText("Get Notified")
.setMessageText("Please enable notifications on your device to use Push Notifications.")
.followDeviceOrientation(true)
.setPositiveBtnText("Allow")
.setNegativeBtnText("Cancel")
.setBackgroundColor(Constants.WHITE)
.setBtnBorderColor(Constants.BLUE)
.setTitleTextColor(Constants.BLUE)
.setMessageTextColor(Constants.BLACK)
.setBtnTextColor(Constants.WHITE)
.setBtnBackgroundColor(Constants.BLUE)
.build()
cleverTapAPI.promptPushPrimer(builder)
Push Primer using Alert InApp
You can use this template to send a basic push primer notification with a title, message, and two buttons.
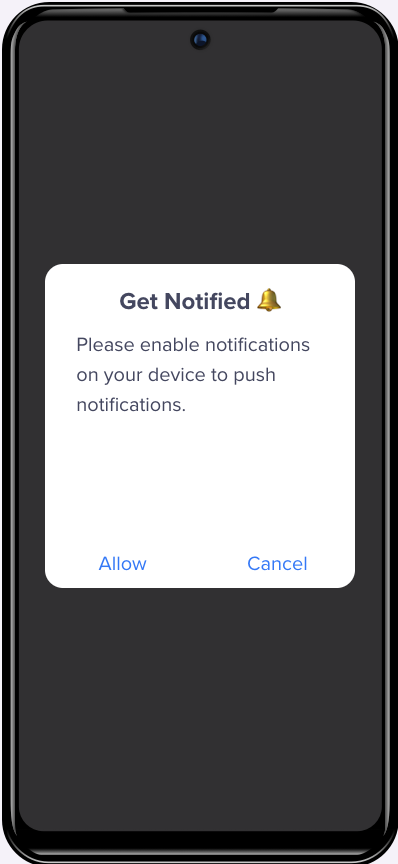
Push Primer Using Alert InApp
Use the following to create your template:
JSONObject jsonObject = CTLocalInApp.builder()
.setInAppType(CTLocalInApp.InAppType.ALERT)
.setTitleText("Get Notified")
.setMessageText("Enable Notification permission")
.followDeviceOrientation(true)
.setPositiveBtnText("Allow")
.setNegativeBtnText("Cancel")
.build();
cleverTapAPI.promptPushPrimer(jsonObject);
val builder = CTLocalInApp.builder()
.setInAppType(CTLocalInApp.InAppType.ALERT)
.setTitleText("Get Notified")
.setMessageText("Enable Notification permission")
.followDeviceOrientation(true)
.setPositiveBtnText("Allow")
.setNegativeBtnText("Cancel")
.build()
cleverTapAPI.promptPushPrimer(builder)
Method Description
The following is a description of all the methods used to create In-App templates.
setInAppType(InAppType)
//Required param
//InAppType - CTLocalInApp.InAppType.HALF_INTERSTITIAL & CTLocalInApp.InAppType.ALERT
//Description - Accepts only HALF_INTERSTITIAL & ALERT type to display the type of InApp.
setFallbackToSettings(Boolean)
//Optional param
//Boolean - true/false
//Description - If true and the permission is denied then we fallback to app’s notification settings, if it’s false then we just throw a verbose log saying permission is denied.
setTitleText(String)
//Required param
//String - Text
//Description - Sets the title of the local InApp.
setMessageText(String)
//Required param
//String - Text
//Description - Sets the subtitle of the local InApp.
followDeviceOrientation(Boolean)
//Required param
//Boolean - true/false
//Description - If true then the local InApp is shown for both portrait and landscape. If it sets false then local InApp only displays for portrait mode.
setPositiveBtnText(String)
//Required param
//String - Text
//Description - Sets the text of the positive button.
setNegativeBtnText(String)
//Required param
//String - Text
//Description - Sets the text of the negative button.
setBackgroundColor(String)
//Optional param
//String - Accepts Hex color as String
//Description - Sets the background color of the local InApp.
setBtnBorderColor(String)
//Optional param
//String - Accepts Hex color as String
//Description - Sets the border color of both positive/negative buttons.
setTitleTextColor(String)
//Optional param
//String - Accepts Hex color as String
//Description - Sets the title color of the local InApp.
setMessageTextColor(String)
//Optional param
//String - Accepts Hex color as String
//Description - Sets the sub-title color of the local InApp.
setBtnTextColor(String)
//Optional param
//String - Accepts Hex color as String
//Description - Sets the color of text for both positive/negative buttons.
setBtnBackgroundColor(String)
//Optional param
//String - Accepts Hex color as String
//Description - Sets the background color for both positive/negative buttons.
setBtnBorderRadius(String)
//Optional param
//String - String
//Description - Sets the radius for both positive/negative buttons. Default radius is “2” if not set.
Call Android System Permission Flow from Web Interface (All types of HTML InApp)
The following two public methods are available to call the Android system permission flow from HTML InApp:
promptPushPermission(boolean shouldShowFallbackSettings)
- Used to trigger OS notification dialog.dismissInAppNotification()
- Used to dismiss the current InApp.
The following is the sample code to request permission on button clicks for HTML campaigns:
<script>
document.querySelector('#bt_gnp').addEventListener(
'click',e => {
if(window.CleverTap){
CleverTap.promptPushPermission(true); // true/false on whether to show app’s notification page if permission is denied.
}
})</script>
Updated 4 months ago