iOS In App Notification
In-App Notifications
The CleverTap SDK allows you to show In-App notifications to your users and it provides the capability to send in-app messages with images, GIFs, video, and audio. You can design in-app notifications right from the dashboard, without writing a single line of code. There is no code required for this feature. You can also do A/B testing on your in-apps.
Note: not applicable inside App Extensions and watchOS apps.
InApp Notification Button onClick Callbacks
iOS SDK v3.7.1 and above supports callback on the click of InApp Notification Buttons by returning a Map of Key-Value pairs. To use this, make sure your class implements the CleverTapInAppNotificationDelegate
and use the following method to handle user-selected actions from InApp notifications:
- (void)inAppNotificationButtonTappedWithCustomExtras:(NSDictionary *)customExtras {
NSLog(@"inAppNotificationButtonTappedWithCustomExtras:%@", customExtras);
...
}
func inAppNotificationButtonTapped(withCustomExtras customExtras: [AnyHashable : Any]!) {
print("In-App Button Tapped with custom extras:", customExtras ?? "");
}
Control In-App Notifications
For CleverTap iOS SDK 3.10.0 and above, you can suspend, discard, or resume in-app notifications.
Note
The CleverTap SDK automatically resumes displaying the in-app notification after every new session.
You can control the in-app notifications in the following ways:
Suspend
The suspendInAppNotifications
method suspends and saves in-app notifications until the resumeInAppNotifications
method is called for the current session.
CleverTap.sharedInstance()?.suspendInAppNotifications()
#import <CleverTapSDK/CleverTap+InAppNotifications.h>
[[CleverTap sharedInstance] suspendInAppNotifications];
Discard
The discardInAppNotifications
method discards in-app notifications until the resumeInAppNotifications
method is called for the current session.
CleverTap.sharedInstance()?.discardInAppNotifications()
#import <CleverTapSDK/CleverTap+InAppNotifications.h>
[[CleverTap sharedInstance] discardInAppNotifications];
Resume
The resumeInAppNotifications
method resumes displaying in-app notifications.
If you call this method after the discardInAppNotifications() method, it resumes the in-app notifications for events raised after the call is performed.
However, if you call the resumeInAppNotifications method after the suspendInAppNotifications() method, then it displays all queued in-app notifications and also resumes in-app notifications for events raised after the call is performed.
CleverTap.sharedInstance()?.resumeInAppNotifications()
#import <CleverTapSDK/CleverTap+InAppNotifications.h>
[[CleverTap sharedInstance] resumeInAppNotifications];
Note
The
showInAppNotificationIfAny
method will be deprecated soon. Use theresumeInAppNotifications
method to manually display the pending in-app notifications.
Custom Handling Deeplink
For CleverTap iOS SDK 3.10.0 and above, you can implement custom handling for URLs of in-app notification CTAs, push notifications, and app Inbox messages.
Check that your class conforms to the CleverTapURLDelegate
protocol by first calling setURLDelegate
. Use the following protocol method shouldHandleCleverTap(_ : forChannel:)
to handle URLs received from channels such as in-app notification CTAs, push notifications, and app Inbox messages:
// Set the URL Delegate
CleverTap.sharedInstance()?.setUrlDelegate(self)
// CleverTapURLDelegate method
public func shouldHandleCleverTap(_ url: URL?, for channel: CleverTapChannel) -> Bool {
print("Handling URL: \(url!) for channel: \(channel)")
return true
}
#import <CleverTapSDK/CleverTapURLDelegate.h>
// Set the URL Delegate
[[CleverTap sharedInstance]setUrlDelegate:self];
// CleverTapURLDelegate method
- (BOOL)shouldHandleCleverTapURL:(NSURL *)url forChannel:(CleverTapChannel)channel {
NSLog(@"Handling URL: \(%@) for channel: \(%d)", url, channel);
return YES;
}
Javascript support in In-App Notifications
CleverTap iOS SDK v3.5.0 and above supports embedding Javascript code inside Custom In-Apps. To make sure your Javascript code works on the app, while creating the InApp campaign, select the checkbox for Enabling javascript during the In-App campaign creation
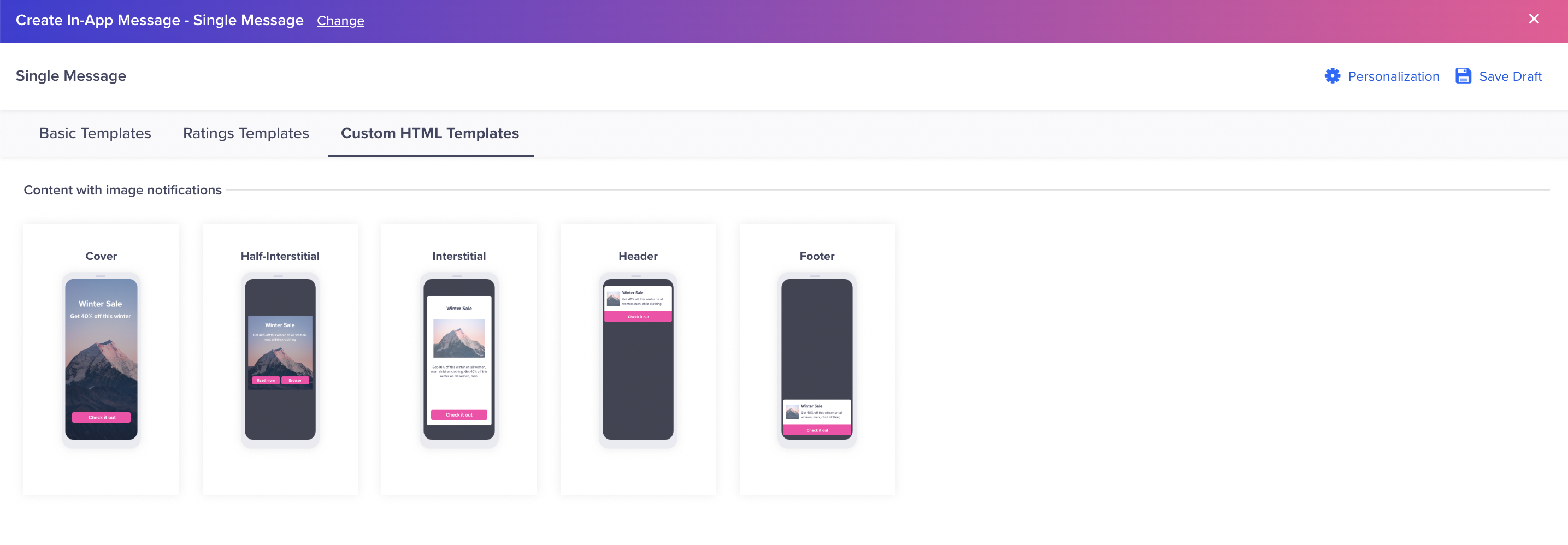
Custom HTML Templates in In-App Notification
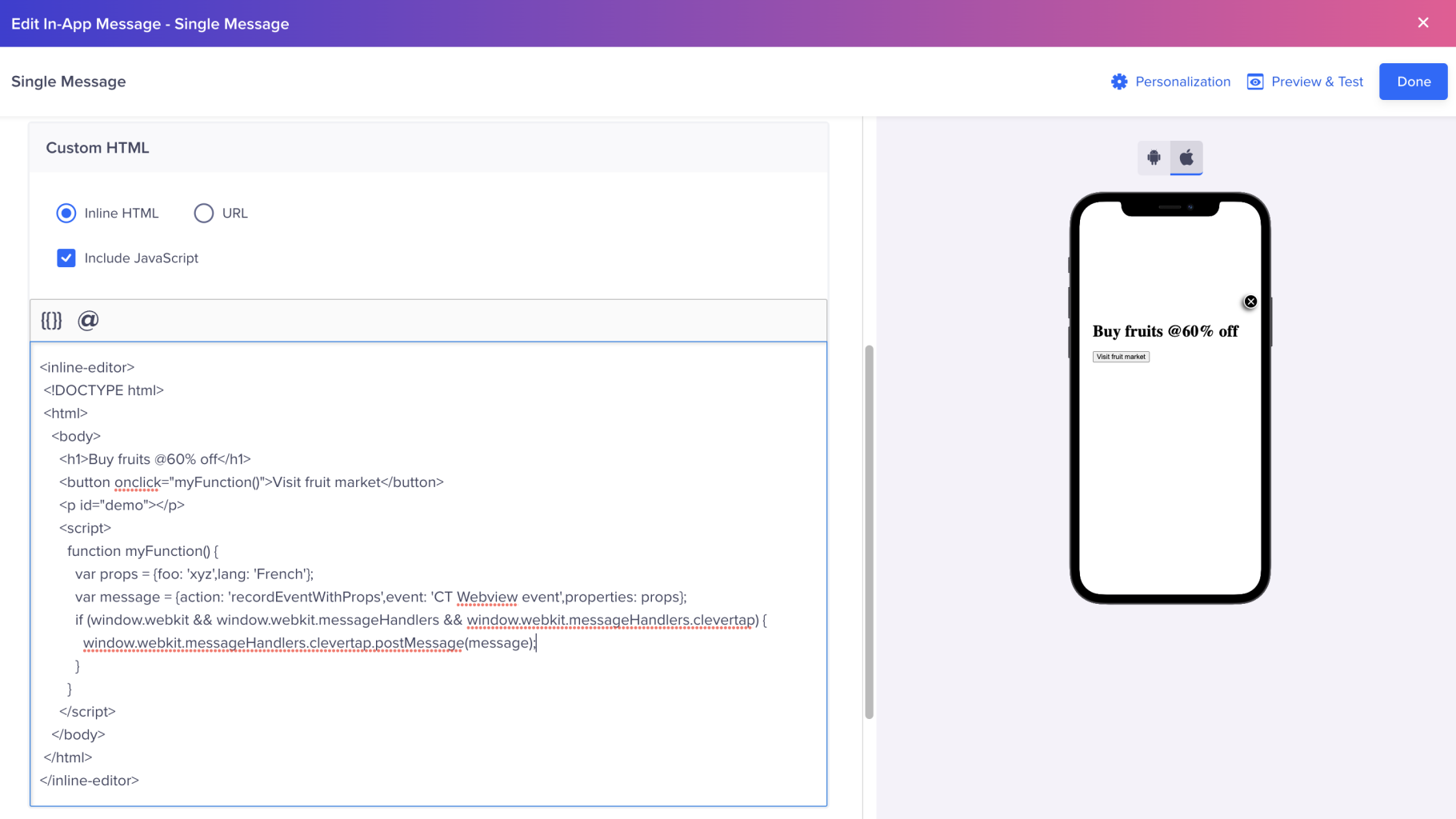
Include JavaScript in In-App Notifications
All methods are explained with examples below:
//Recording a User Event called Product Viewed with properties, in JS enabled custom In-Apps
var props = {foo: 'xyz', lang: 'French'};
var message = { action:'recordEventWithProps', event:'Product Viewed', properties: props};
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Updating profile properties of the User in JS enabled custom In-Apps
const props = { name: 'John', email: 'john@mymail.com' , score: 0};
const message = { action:'profilePush', properties: props };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Adding user property for the User in JS enabled custom In-Apps
const message = { action: 'profileAddMultiValue', value: 'coat', key: 'myStuff' };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Adding multiple user properties for the User in JS enabled custom In-Apps
const message = { action: 'profileAddMultiValues', values: ['bag', 'kitkat'], key: 'myStuff' };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Removing a unique value from a multi-value profile in JS enabled custom In-Apps
const message = { action: 'profileRemoveMultiValue', value:'scarf', key: 'myStuff' };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Removing multiple unique values from a multi-value profile in JS enabled custom In-Apps
const message = { action: 'profileRemoveMultiValues', values:['scarf', 'knife'], key: 'myStuff' };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Removing a user property by specifying a key in JS enabled custom In-Apps
const message = { action: 'profileRemoveValueForKey', key: 'myStuff' };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Setting a user property by specifying the key in JS enabled custom In-Apps
const message = { action: 'profileSetMultiValues', values:['scarf', 'knife'], key: 'myStuff' };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
// Increment user property value by specifying the key in JS enabled custom In-Apps
const message = { action: 'profileIncrementValueBy', value: 1, key: 'score' };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
// Decrement user property value by specifying the key in JS enabled custom In-Apps
const message = { action: 'profileDecrementValueBy', value: 1, key: 'score' };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Calling onUserLogin
const props = { name: 'JohnWeb', email: 'johnWeb@mymail.com' };
const message = { action:'onUserLogin', properties: props };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Recording a Charged Event in JS enabled custom In-Apps
const chargeDetails = { "Amount" : 300, "Payment mode": "Credit Card", "Charged ID": 24052013 };
const items = [{"Category": "books","Book name": "The Millionaire next door","Quantity": 1},
{"Category": "books","Book name": "The Millionaire previous door","Quantity": 5}];
const message = { action:'recordChargedEvent', chargeDetails: chargeDetails, items: items };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
//Prompt for push permission
const message = { action:'promptForPushPermission', showFallbackSettings:true };
if (window.webkit && window.webkit.messageHandlers && window.webkit.messageHandlers.clevertap) {
window.webkit.messageHandlers.clevertap.postMessage(message);
}
Inline Media
To add inline media support in custom HTML for in-app notifications, add the key playsinline with value as 1 or true as a query parameter in your media URL. For example, watch this YouTube video. This functionality is available for CleverTap iOS SDK 3.7.2 and above.
Updated 3 months ago