Android Advanced Features
Learn more about advanced Android features.
Overview
Add advanced features to your app.
Opt Out
From CleverTap Android SDK 3.1.9 onwards, in compliance with GDPR, we provide the following method using which the app can stop sending events to CleverTap.
cleverTap.setOptOut(true);
cleverTap.setOptOut(true)
If the user wants to opt in again to share their data, you call the same method like this.
cleverTap.setOptOut(false);
cleverTap.setOptOut(false)
Enable Device Networking Info Reporting
From CleverTap Android SDK 3.1.9 onwards, in compliance with GDPR, the CleverTap Android SDK will not capture any kind of personal information like WiFi, Bluetooth, Network Information and user IP information. To enable the capturing of all this information, you can call the following method
cleverTap.enableDeviceNetworkInfoReporting(true);
cleverTap.enableDeviceNetworkInfoReporting(true)
To disable Device Network information to be sent to CleverTap you can use the same method as follows.
cleverTap.enableDeviceNetworkInfoReporting(false);
cleverTap.enableDeviceNetworkInfoReporting(false)
By default, CleverTap does not collect device network information.
Set Offline
CleverTap Android SDK provides a method to set the user as offline. Setting the user offline means that no events/profile information will be sent to CleverTap. You can set the user as offline using the following method
cleverTap.setOffline(true);
cleverTap.setOffline(true)
You can set the user back online using the following method
cleverTap.setOffline(false);
cleverTap.setOffline(false)
Encryption of PII Data
PII data is stored across the SDK and could be sensitive information. From CleverTap SDK v5.2.0 onwards, you can enable encryption for PII data such as Email, Identity, Name, and Phone.
Currently, two levels of encryption are supported, i.e., None(0) and Medium(1). The encryption level is None by default.
- None: All stored data is in plaintext
- Medium: PII data is encrypted completely
The only way to set the encryption level for the default instance is from the AndroidManifest.xml.
Add the following to the AndroidManifest.xml file:
<meta-data
android:name="CLEVERTAP_ENCRYPTION_LEVEL"
android:value="1" />
Different instances can have different encryption levels. To set an encryption level for an additional instance:
CleverTapInstanceConfig clevertapAdditionalInstanceConfig = CleverTapInstanceConfig.createInstance(
applicationContext,
"ADDITIONAL_CLEVERTAP_ACCOUNT_ID",
"ADDITIONAL_CLEVERTAP_ACCOUNT_TOKEN"
)
clevertapAdditionalInstanceConfig.setEncryptionLevel(CryptHandler.EncryptionLevel.MEDIUM)
CleverTapAPI clevertapAdditionalInstance = CleverTapAPI.instanceWithConfig(applicationContext ,clevertapAdditionalInstanceConfig)
Referral Tracking
From CleverTap SDK v3.6.4 onwards, add the following Gradle dependency to capture UTM details, app-install time, referrer click time, and other metrics provided by Google Install Referrer Library.
implementation 'com.android.installreferrer:installreferrer:2.2'
Debugging
Follow the steps for debugging your app:
- By default, CleverTap logs are set to CleverTap.Loglevel.INFO. We recommend that you set the SDK to
VERBOSE
mode to log warnings or other important messages to the Android logging system during development. This can be done by setting the debug level toCleverTap.Loglevel.VERBOSE
. If you want to disable CleverTap logs for the production environment, you can set the debug level toCleverTap.Loglevel.OFF
.
CleverTapAPI.setDebugLevel(CleverTapAPI.LogLevel.INFO); //Default Log level
CleverTapAPI.setDebugLevel(CleverTapAPI.LogLevel.DEBUG); //Set Log level to DEBUG log warnings or other important messages
CleverTapAPI.setDebugLevel(CleverTapAPI.LogLevel.VERBOSE); //Set Log level to VERBOSE
CleverTapAPI.setDebugLevel(CleverTapAPI.LogLevel.OFF); //Switch off logs for Production environment
CleverTapAPI.setDebugLevel(CleverTapAPI.LogLevel.INFO) //Default Log level
CleverTapAPI.setDebugLevel(CleverTapAPI.LogLevel.DEBUG) //Set Log level to DEBUG log warnings or other important messages
CleverTapAPI.setDebugLevel(CleverTapAPI.LogLevel.VERBOSE) //Set Log level to VERBOSE
CleverTapAPI.setDebugLevel(CleverTapAPI.LogLevel.OFF) //Switch off logs for Production environment}
- After setting the debug level to
CleverTap.Loglevel.VERBOSE
, search for Clevertap. The logcat displays the handshakes between CleverTap and your app. - Copy the CleverTap ID from the logs.
- Open the CleverTap dashboard.
- Navigate to Segments> Find People > search By Identity.
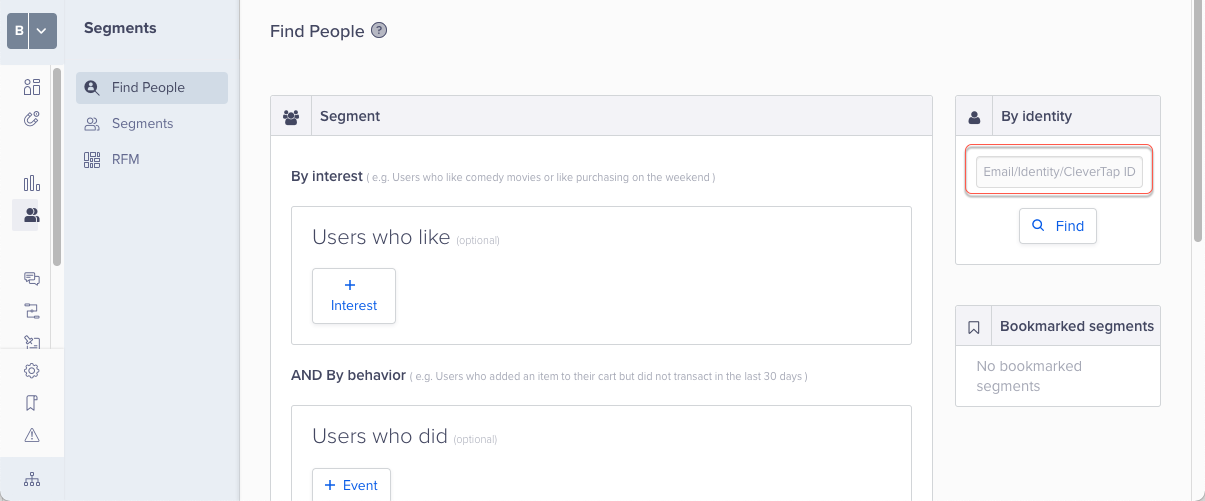
Find People by Identity
Changing Account Credentials
If youβd not want to insert your account credentials in your appβs AndroidManifest.xml, or would like to change your account ID programmatically, you need to create a custom Application class (if you donβt have one already) with the following content.
import android.app.Application;
import com.clevertap.android.sdk.ActivityLifecycleCallback;
import com.clevertap.android.sdk.CleverTapAPI;
public class MyApplication extends Application {
@Override
public void onCreate() {
CleverTapAPI.changeCredentials("Your account ID here", "Your account token here");
ActivityLifecycleCallback.register(this); // Must be called before super.onCreate()
super.onCreate();
}
}
import android.app.Application
import com.clevertap.android.sdk.ActivityLifecycleCallback
import com.clevertap.android.sdk.CleverTapAPI
class MyApplication:Application() {
override fun onCreate() {
CleverTapAPI.changeCredentials("Your account ID here", "Your account token here")
ActivityLifecycleCallback.register(this) // Must be called before super.onCreate()
super.onCreate()
}
}
If youβve just created this subclass of Application, update your AndroidManifest.xml to specify your subclass.
<application
android:label="@string/app_name"
android:icon="@drawable/ic_launcher"
android:name=".MyApplication">
If you have used this method, to set your CleverTap Account ID and Token, please do not add these values to your manifest file.
Manually Updating User Location
The application is responsible for requesting the userβs permission to use location. If this permission is granted, location can be passed to CleverTap.
The App can pass the location to the SDK using setLocation.
CleverTapAPI cleverTapAPI = CleverTapAPI.getDefaultInstance(getApplicationContext());
cleverTapAPI.setLocation(location); //android.location.Location
val cleverTapAPI = CleverTapAPI.getDefaultInstance(getApplicationContext())
cleverTapAPI.setLocation(location) //android.location.Location}
Using Your Existing Activity Lifecycle Listener
If youβd like to use your own activity lifecycle listener, please update it to incorporate the following code.
application.registerActivityLifecycleCallbacks(
new android.app.Application.ActivityLifecycleCallbacks() {
@Override
public void onActivityCreated(Activity activity, Bundle bundle) {
CleverTapAPI.setAppForeground(true);
try {
CleverTapAPI.getDefaultInstance(application).pushNotificationClickedEvent(activity.getIntent().getExtras());
} catch (Throwable t) {
// Ignore
}
try {
Intent intent = activity.getIntent();
Uri data = intent.getData();
CleverTapAPI.getDefaultInstance(application).pushDeepLink(data);
} catch (Throwable t) {
// Ignore
}
}
@Override
public void onActivityStarted(Activity activity) {
}
@Override
public void onActivityResumed(Activity activity) {
try {
CleverTapAPI.getDefaultInstance(application).onActivityResumed(activity);
} catch (Throwable t) {
// Ignore
}
}
@Override
public void onActivityPaused(Activity activity) {
try {
CleverTapAPI.getDefaultInstance(application).onActivityPaused();
} catch (Throwable t) {
// Ignore
}
}
@Override
public void onActivityStopped(Activity activity) {
}
@Override
public void onActivitySaveInstanceState(Activity activity, Bundle bundle) {
}
@Override
public void onActivityDestroyed(Activity activity) {
}
}
);
registerActivityLifecycleCallbacks(object : android.app.Application.ActivityLifecycleCallbacks {
override fun onActivityCreated(activity: Activity, savedInstanceState: Bundle?) {
CleverTapAPI.setAppForeground(true)
try
{
CleverTapAPI.getDefaultInstance(applicationContext)?.pushNotificationClickedEvent(activity.intent.extras)
}
catch (t:Throwable) {
// Ignore
}
try
{
val intent = activity.intent
val data = intent.data
CleverTapAPI.getDefaultInstance(applicationContext)?.pushDeepLink(data)
}
catch (t:Throwable) {
// Ignore
}
}
override fun onActivityStarted(activity: Activity) {
}
override fun onActivityResumed(activity: Activity) {
try
{
CleverTapAPI.onActivityResumed(activity)
}
catch (t:Throwable) {
// Ignore
}
}
override fun onActivityPaused(activity: Activity) {
try
{
CleverTapAPI.onActivityPaused()
}
catch (t:Throwable) {
// Ignore
}
}
override fun onActivityStopped(activity: Activity) {
}
override fun onActivitySaveInstanceState(activity: Activity, outState: Bundle){
}
override fun onActivityDestroyed(activity: Activity) {
}
})
Update on Google Play Store privacy requirements
Refer to Google play store privacy requirements.
Updated about 1 year ago