Signed Call™ Web SDK
Learn how to integrate Signed Call™ Web SDK into your website and CRM portal.
Overview
CleverTap provides in-app calls via its Signed Call™ Web SDK, which means you can make and receive calls on any webpage or device with an internet connection and the Signed Call Web SDK. This document shows you how to integrate the Signed Call Web SDK and manage calls. To know more about the Signed Call feature, refer to Signed Call.
Permission Requirements
Following are the basic permission requirements for the Signed Call Web SDK:
- Microphone (Required)
- Notification (Optional but recommended)
Browser Requirements
Following are the basic browser requirements for the Signed Call Web SDK to work:
Browser Name | Version Number |
---|---|
Google Chrome | V55 and above |
Microsoft Edge | V15 and above |
Mozilla Firefox | V43 and above |
Opera | V43 and above |
Safari | Does not support |
Integrate the CleverTap Web SDK
The Signed Call Web SDK uses CleverTap SDK for analytics. Signed Call Web SDK requires an active CleverTap instance as a parameter during the SDK initialization. If the CleverTap instance is unavailable, the Signed Call Web SDK returns an error ERR_MISSING_INITPARAMETERS
, and the Signed Call Web SDK does not initialize.
To integrate the CleverTap Web SDK, refer to the CleverTap Web SDK Integration Guide.
Minimum Supported Version
The Signed Call Web SDK integrates with CleverTap SDK v1.2.0 or higher.
Integrate Signed Call Web SDK
You can integrate Signed Call Web SDK using the following:
NPM
To integrate the Signed Call Web SDK using NPM, you can add the Signed Call Web SDK as an npm
to your web app.
Use the following npm
command to install the package:
npm install clevertap-signed-call
Import the installed package as follows:
import {initSignedCall} from 'clevertap-signed-call'
CDN
To integrate the Signed Call Web SDK using CDN, use the following CDN
link:
<https://d2r1yp2w7bby2u.cloudfront.net/js/clevertap-signedcall.umd.js>
Include the above CDN link in the script
tag as shown below:
<script src="https://d2r1yp2w7bby2u.cloudfront.net/js/clevertap-signedcall.umd.js"></script>
Initialize and Authenticate Signed Call Web SDK
Create a new instance of a SignedCallClient
by calling the initSignedCall()
function. This function returns a promise
object whose then()
and catch()
can be utilized for the following scenarios:
Scenario 1: If the promise resolves successfully, the then()
method receives SignedCallClient
as a response, meaning that the Signed Call Web SDK is initialized successfully.
Scenario 2: If the promise is rejected, the catch()
method receives an error, meaning that the Signed Call Web SDK initialization failed.
The syntax for initializing and authenticating the Signed Call Web SDK using NPM is as follows:
let SignedCallClient
initSignedCall(
{
accountId: <string>,
apikey: <string>,
cuid: <string>,
clevertap: <clevertap sdk instance>,
name: <string / optional>,
ringtone: <string / optional>
}
).then(client => SignedCallClient = client).catch(err => console.log(err))
The syntax for initializing and authenticating the Signed Call Web SDK using CDN is as follows:
SignedCallSDK.initSignedCall({
accountId: <string>,
apikey: <string>,
cuid: <string>,
clevertap: <clevertap sdk instance>,
name: <string / optional>,
ringtone: <string / optional>
})
.then((client) => {
console.log({ client })
SignedCallClient = client;
})
.catch(e => console.log(e));
}
The options parameter in the initSignedCall
function expects a JSON object with the following properties:
Properties | Description | Type | Notes |
---|---|---|---|
|
| String | Required |
|
| String | Required |
| Unique identity of the user. | String | Required |
| The CleverTap Web SDK instance. | Object | Required |
|
| String | Optional |
ringtone | The URL of the ringtone played on the caller's end during the outgoing call. Note: The default ringtone plays without this parameter. | String | Optional |
CUID Validation Rules
The following are the validation rules for
cuid
:
- Must range between 5 and 50 characters.
- Must start with either alphabet or number.
- The name is case-sensitive, and only '_' is allowed as a special character.
- The
cuid
parameter cannot be of type number-number, that is, a number followed by a special character, which is again followed by another number. For example, org_25 is allowed, but 91_8899555 is not permitted.- Must be unique for every user.
- Must be unique for every device to allow multiple logins for the user from different devices. In such cases, the user will have multiple
cuid
s. If the same CUID is associated with multiple devices, the device with the most recent SignedCall initialization is prioritized for receiving the call.
Network Reconnection
In case of network reconnection, the Signed Call Web SDK might take some time to reinitialise. This duration ranges from 30 seconds to 6 minutes, depending on the network quality.
Make a Signed Call
The dialing screen displays when the SignedCallClient
from the init()
function invokes the call()
method to make an outbound call.
This method returns a promise
object whose then()
and catch()
method can be utilized for the following scenarios:
Scenario 1: When the call is answered, the outgoing call screen transitions into the ongoing call screen. After the transition, the then()
method receives an over
status and indicates that the call is completed successfully.
Scenario 2: The catch()
method receives three status types: declined
, missed
, and cancelled
. Each status indicates a different scenario: declined
means the receiver rejected the call, missed
indicates the call was not answered, and cancelled
can occur when the caller cancels the call or the SignedCall system automatically cancels the call after 35 seconds of no response, which should be treated as equivalent to no answer.
Note
In the given scenarios (Scenario 1 and Scenario 2), the SignedCall system offers various call states. These call states, including "over," "missed," "declined," and "cancelled," serve as valuable indicators for managing appropriate call statuses in your application
The call()
parameters are as follows:
Parameters | Description | Type | Notes |
---|---|---|---|
| It is the receiver's | String | Required |
| It specifies the context of the call. For example, Trainer is calling, Grocer is calling, Tutor is calling, and so on. | String | Required |
| It is a JSON object with the following properties:
| Object | Optional |
Call Button Handling
Before initiating the call, verify whether the Signed Call services are enabled. Use the following isEnabled()
method that returns Boolean data type:
if (SignedCallClient.isEnabled()) {
SignedCallClient.call(receiver, context, callOptions).then(response => {
// if the call has been answered
console.log("call status is: ",response)
}).catch (err => {
// if the call is either missed or declined
console.log("call status is: ", err)
})
}
Busy Handling
Signed Call Web SDK smartly handles the scenarios when the user is busy on a call.
The following scenarios describe the Signed Call Web SDK behavior:
Scenario : The user is busy on a call (VoIP), and another user initiates a VoIP call to the busy user. In this case, the Signed Call Web SDK provides a console message User is busy on another call and declines the initiated call. The customer can show custom messages on their web interface when the user is busy on another call.
Call Hangup Functionality
The hangup()
function ends an ongoing call. The hangup functionality is user-driven, and ending the call depends on the user. For example, if one of the users in a call clicks the call hangup button from the ongoing call screen, the Signed Call Web SDK calls the hangup()
function internally to end the call.
In the case of a metered call, when a business wants to end the call after a specific duration, it must maintain a timer in the app and call the SignedCallClient.hangup()
function at the appropriate time.
SignedCallClient.hangup()
Logout the Signed Call Web SDK
The logout function disables the Signed Call Web SDK's calling feature by calling the SignedCallClient.logout()
method. After calling the logout()
function, you must repeat the Initialization and Authentication steps to make a new call.
SignedCallClient.logout()
Manage Notification Permission
To receive a Signed Call notification from the web, you must enable both system-level and browser-level permissions. If any one of the permission is not enabled, you will not receive the signed call notification on the browser.
System-level Permissions
You can enable this permission from the Notification Center of your system. The following sections provide information about enabling permission from Macbook and Windows systems.
MacBook
For Mac, toggle ON the Allow Notifications option from the Notifications Center to enable the notification for the required web browser, as shown in the following figure:
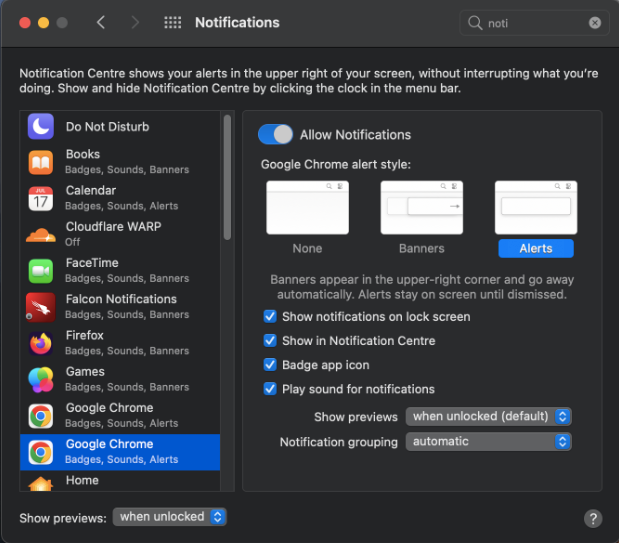
Enable Notification Permission for Google Chrome on Macbook
Windows
For Windows, toggle ON the Notifications option from the Notifications Settings to enable the notifications, as shown in the following figure:
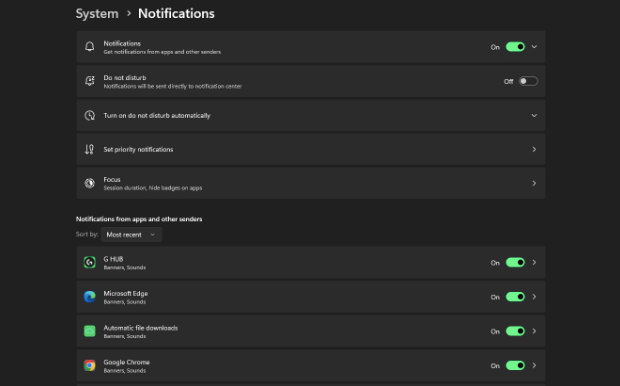
Enable Notifications for Google Chrome on Windows
Browser-level Permissions
Whenever the Signed Web SDK is initialized, you are prompted to provide permission to receive the Signed Call notifications from the Web, as shown in the following figure. Click Allow to enable browser-level notification.
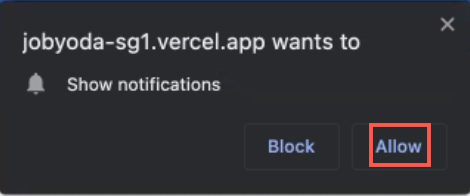
Allow Browser Level Permissions
The following is the sample Signed Call notification displayed on Google Chrome:
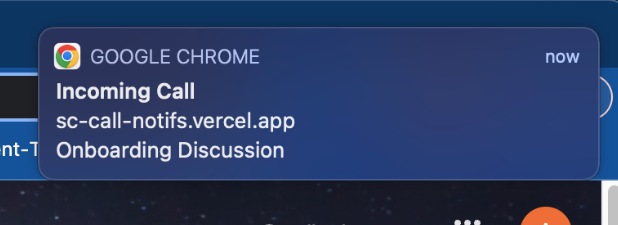
Sample Notification in Google Chrome
Notifications in Do Not Disturb (DND) Mode
If the user has enabled DND mode, the notification is sent directly to the Notification Center and rendered only in the notification tray.
Example
The following example describes the implementation of Signed Call Web SDK Initialization, Make Call, Hangup Call (for metered connection), and Logout functions of the clevertap-signed-call
via Node Package Manager (NPM):
import {initSignedCall} from 'clevertap-signed-call'
//initiate the sdk
initSignedCall({
accountId, //string, required
apikey, // string, required
cuid, // string,required
clevertap, // clevertap instance, required
name, // string, optional
ringtone // string, optional
}).then(client => SignedCallClient = client).catch(err => console.error(err))
// make a call
function call() {
let callOptions = {
receiver_image: "", // optional, string
initiator_image: "" // optional, string
};
/**
* receiver {string, required}: cuid whom you are calling
* context {reason, required}: reason of call
* callOptions {optional}
* */
if (SignedCallClient.isEnabled()) {
SignedCallClient.call(receiver, context,calloptions)
.then((response) => {
console.log("Call response : ", response);
})
.catch((error) => {
console.error(error);
})
} else {
// wait for sdk to be enabled or re-initiate the sdk
}
}
// Hangup a call automatically after 20000ms
setTimeout((SignedCallClient.hangup()), 20000)
// Logout the sdk
let logout = function () {
SignedCallClient.logout();
}
The following example describes the implementation of Signed Call Web SDK Initialization, Make Call, Hangup Call (for metered connection), and Logout functions via CDN.
<script src="https://d2r1yp2w7bby2u.cloudfront.net/js/clevertap-signedcall.umd.js"></script>
//initiate the sdk
<script type="text/javascript">
SignedCallSDK.initSignedCall({
accountId: accountId,
apikey: apikey,
// cc: cc,
// phone: phone,
cuid: cuid,
name: name,
clevertap
})
.then((client) => {
console.log({ client })
SignedCallClient = client;
hide("connectBtn");
show("disconnectBtn");
show("hr");
show("dialler");
})
.catch(e => console.log(e));
}
// make a call
function call() {
let callOptions = {
receiver_image: "", // optional, string
initiator_image: "" // optional, string
};
/**
* receiver {string, required}: cuid whom you are calling
* context {reason, required}: reason of call
* callOptions {optional}
* */
if (SignedCallClient.isEnabled()) {
SignedCallClient.call(receiver, context,calloptions)
.then((response) => {
console.log("Call response : ", response);
})
.catch((error) => {
console.error(error);
})
} else {
// wait for sdk to be enabled or re-initiate the sdk
}
}
// Hangup a call automatically after 20000ms
setTimeout((SignedCallClient.hangup()), 20000)
// Logout the sdk
let logout = function () {
SignedCallClient.logout();
}
</script>
Errors
The following table lists the different errors and their probable causes:
Error | Reason |
---|---|
ERR_MISSING_INITPARAMETERS | One (or more) mandatory parameter is missing in SDK Initialization and Authentication. |
ERR_INVALID_INITPARAMETERS | Parameters are not valid. |
ERR_MISSING_CT_ACCOUNTID | Signed Call Web SDK is unable to find the Account ID associated with CleverTap. |
ERR_MISSING_CT_ID | Signed Call Web SDK cannot find the CleverTap ID. |
ERR_INVALID_CREDENTIALS | The Account ID or API Key provided to the Signed Call is incorrect. |
ERR_ALREADY_SIGNEDIN | The cuid is currently connected to another device. |
ERR_MIC_UNAVAILABLE | Microphone permission denied. |
ERR_OUTGOING_CALL_IN_PROGRESS | If a call is already in progress, then another can only be initiated once the current call is over, missed, declined, or canceled. |
ERR_INVALID_CALL_PARAMETERS | The parameters provided in Make Call are incorrect. |
ERR_INTERNET_LOST | The call did not occur successfully due to the loss of internet connection. |
404 | The receiver's cuid is offline. |
FAQ
Q. Are Signed Call accountId
and apikey
the same as CleverTap's accountId
and token
?
accountId
and apikey
the same as CleverTap's accountId
and token
?A. No. Signed Call accountId
and apikey
differ from CleverTap's accountId
and token
. You can find these details under your dashboard's Signed Call Settings.
Q. How to resolve ERR_MISSING_CT_ID
error even after passing the correct CleverTap instance to the clevertap-signed-call
?
ERR_MISSING_CT_ID
error even after passing the correct CleverTap instance to the clevertap-signed-call
?A. This error may occur if:
- The CleverTap SDK's
region
andaccountId
parameters are incorrect. - CleverTap SDK is not initialized.
Ensure that these details are correct, and if this issue persists, raise an issue at CleverTap Support.
Q. Why is it recommended to enable the web browser notification permission for the business?
A. CleverTap recommends enabling web browser notification permission to avoid missing notifications in the following scenarios:
-
Use Case 1
A user is actively working on the desktop but has minimized the browser. In this case, if the browser notification is not enabled, the user will find it difficult to land on the correct browser tab when receiving a call. -
Use Case 2
A user is actively working on a different browser tab. CleverTap highly recommends enabling the browser notification for the best web experience.
Updated 29 days ago